There are many times we need to adjust widths of children widgets to make them fit to a device’s screen size. If we aren’t sure whether to keep display a widget or to put it into a new row, we can use Wrap
widget.
Wrap widget shows its children in multiple horizontal or vertical runs. It renders it’s children one at a time and puts the next children to the next row automatically if there is no space left in the current row.
Table of Contents
Wrap widget with Chip example
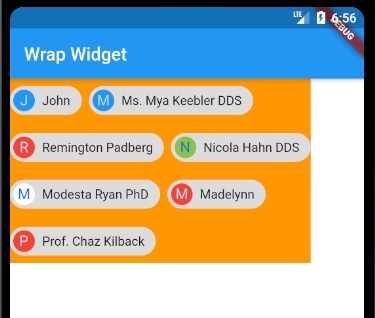
import 'package:flutter/material.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Wrap Widget'), ), backgroundColor: Colors.white, body: SafeArea( child: Container( color: Colors.orange, child: Wrap( spacing: 8.0, // gap between adjacent chips runSpacing: 4.0, // gap between lines children: <Widget>[ MyChip(label: 'John'), MyChip(label: 'Ms. Mya Keebler DDS'), MyChip(label: 'Remington Padberg', color: Colors.red), MyChip(label: 'Nicola Hahn DDS', color: Colors.lightGreen), MyChip(label: 'Modesta Ryan PhD', color: Colors.white), MyChip(label: 'Madelynn', color: Colors.red), MyChip(label: 'Prof. Chaz Kilback', color: Colors.red), ], ), ), ), ); } } class MyChip extends StatelessWidget { final Color color; final String label; const MyChip({Key key, this.color, this.label}) : super(key: key); @override Widget build(BuildContext context) { return Chip( avatar: CircleAvatar(backgroundColor: color ?? Colors.blue, child: Text(label[0])), label: Text(label), ); } }
Wrap Widget Properties
- alignment : Determine whether children should wrap in main axis. Values are WrapAlignment.center, WrapAlignment.end, WrapAlignment.spaceAround, WrapAlignment.spaceBetween, WrapAlignment.spaceEvenly.
- direction : Determine direction like horizontal for Row and vertical for Column.
- spacing : Determine space between each item.
- runSpacing : Determine space between each row like line height.