There are many cool date pickers and calendar libraries for Flutter. The following are the best ones:
Table of Contents
flutter_date_pickers
This package provides day, week, range, and month date pickers.
DayPicker
for one dayWeekPicker
for whole weekRangePicker
for random rangeMonthPicker
for month
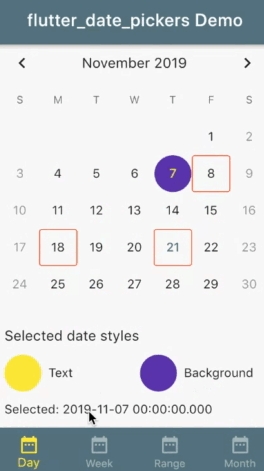
Widget buildWeekDatePicker (DateTime selectedDate, DateTime firstAllowedDate, DateTime lastAllowedDate, ValueChanged<DatePeriod> onNewSelected) { DatePickerRangeStyles styles = DatePickerRangeStyles( selectedPeriodLastDecoration: BoxDecoration( color: Colors.red, borderRadius: BorderRadius.only( topRight: Radius.circular(10.0), bottomRight: Radius.circular(10.0))), selectedPeriodStartDecoration: BoxDecoration( color: Colors.green, borderRadius: BorderRadius.only( topLeft: Radius.circular(10.0), bottomLeft: Radius.circular(10.0)), ), selectedPeriodMiddleDecoration: BoxDecoration( color: Colors.yellow, shape: BoxShape.rectangle), ); return WeekPicker( selectedDate: selectedDate, onChanged: onNewSelected, firstDate: firstAllowedDate, lastDate: lastAllowedDate, datePickerStyles: styles ); }
table_calendar
This is a feature-rich Flutter Calendar with gestures, animations and multiple formats.
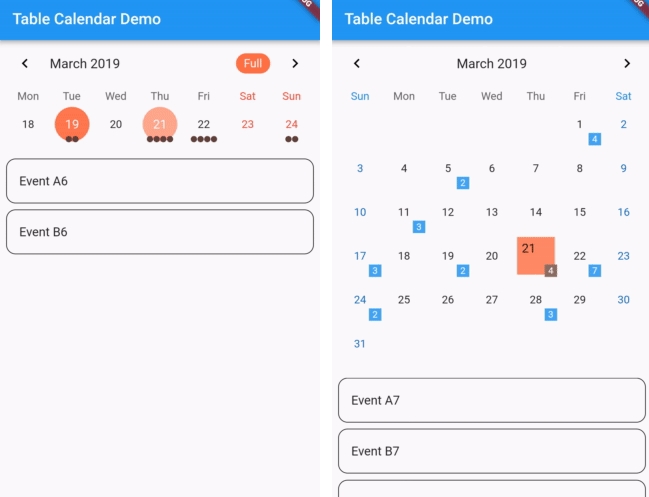
This library has custom builders for developers to create flexible UI, and CalendarController to control any part of the calendar.
The TableCalendar widget only needs the controller to work out of the box.
CalendarController _calendarController = CalendarController(); TableCalendar( calendarController: _calendarController, );
date_picker_timeline
This widget provides a calendar as a horizontal timeline.
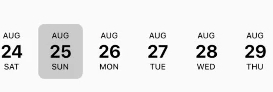
Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ DatePicker( DateTime.now(), initialSelectedDate: DateTime.now(), selectionColor: Colors.black, selectedTextColor: Colors.white, onDateChange: (date) { // New date selected setState(() { _selectedValue = date; }); }, ), ], )
syncfusion_flutter_datepicker
Syncfusion Flutter Date Range Picker allows users to select a single date, multiple dates, or a range of dates easily.
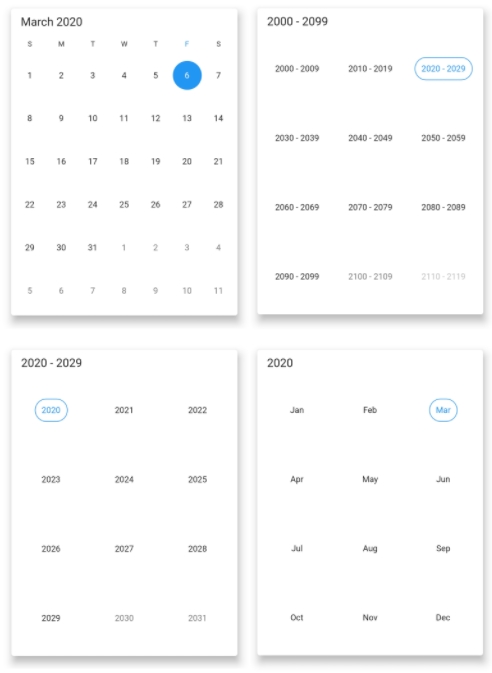
The library offers month, year, decade, and century view options for exact date selection, and minimum, maximum, and disabled dates to restrict date selection.
However, the only con of this package is that it is a commercial one. You need to purchase a license to use it in apps.
time_machine
The Dart Time Machine is a date and time library for Flutter. It supports timezones, calendars, cultures, formatting and parsing.
It works for both the app and the web.
// Sets up timezone and culture information await TimeMachine.initialize(); print('Hello, ${DateTimeZone.local} from the Dart Time Machine!\n'); var tzdb = await DateTimeZoneProviders.tzdb; var paris = await tzdb["Europe/Paris"]; var now = Instant.now(); print('Basic'); print('UTC Time: $now'); print('Local Time: ${now.inLocalZone()}'); print('Paris Time: ${now.inZone(paris)}\n'); print('Formatted'); print('UTC Time: ${now.toString('dddd yyyy-MM-dd HH:mm')}'); print('Local Time: ${now.inLocalZone().toString('dddd yyyy-MM-dd HH:mm')}\n'); var french = await Cultures.getCulture('fr-FR'); print('Formatted and French ($french)'); print('UTC Time: ${now.toString('dddd yyyy-MM-dd HH:mm', french)}'); print('Local Time: ${now.inLocalZone().toString('dddd yyyy-MM-dd HH:mm', french)}\n'); print('Parse French Formatted ZonedDateTime'); // without the 'z' parsing will be forced to interpret the timezone as UTC var localText = now .inLocalZone() .toString('dddd yyyy-MM-dd HH:mm z', french); var localClone = ZonedDateTimePattern .createWithCulture('dddd yyyy-MM-dd HH:mm z', french) .parse(localText); print(localClone.value);
calendar_views
This is a collection of customizable calendar-related widgets for Flutter.
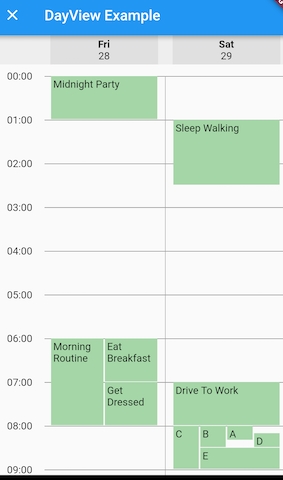
It supports day and month views.