FutureBuilder is a Flutter widget that builds itself based on the latest snapshot of a future operation.
This widget waits for an async function’s result and calls the builder function where we build the widget as soon as the result is produced.
This is an example of how to use FutureBuilder with ListView to obtain and show data from a file.
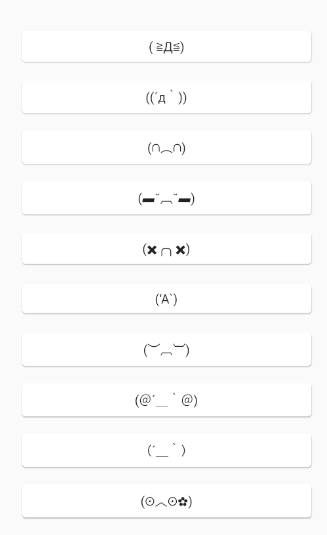
Data call
Future<List<Emoji>> _loadEmojiAsList() async{
final str = rootBundle.loadString('assets/emoji.txt');
final emojis = List<Emoji>();
List<String> lines = LineSplitter.split(str).toList();
lines.forEach((element) {
emojis.add(Emoji(element.trim()));
});
return List<Emoji>();
}
FutureBuilder with ListView
Widget _list() {
return FutureBuilder(
builder: (BuildContext context, AsyncSnapshot<dynamic> snapshot) {
//show progress bar if no data
if (snapshot.connectionState == ConnectionState.none &&
!snapshot.hasData) {
return Text('No emoji');
}
return ListView.builder(
scrollDirection: Axis.vertical,
shrinkWrap: true,
itemCount: snapshot.data.length,
itemBuilder: (context, index) {
return ListTile(
title: Card(
child: Padding(
padding: EdgeInsets.all(8),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(snapshot.data[index].text),
],
))),
);
});
},
future: _loadEmojiAsList(),
initialData: List<Emoji>(),
);
}