Dismissible is a widget that can be dismissed by dragging in the indicated direction. With Dismissible widget, it is as easy to dismiss a widget on your screen as if you were dragging the object away with your finger.
In the example below, the app allows users to swipe items off the list. There is a alert dialog appears to confirm of the deletion.
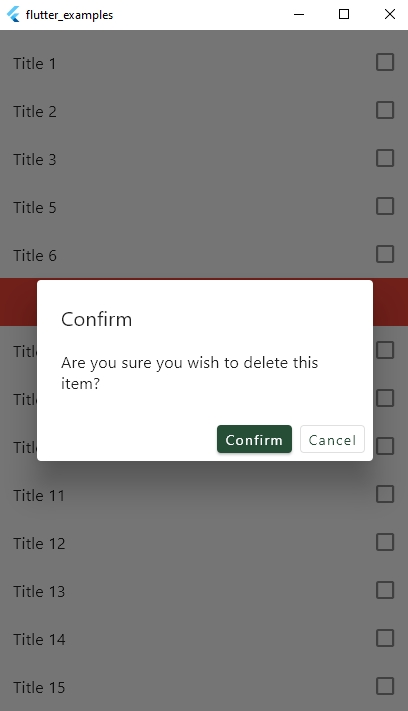
import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> { List<int> items = List<int>.generate(20, (int index) => index); @override Widget build(BuildContext context) { return Scaffold( body: ListView.builder( itemCount: items.length, padding: const EdgeInsets.symmetric(vertical: 8.0), itemBuilder: (BuildContext context, int index) { return Dismissible( key: ValueKey<int>(items[index]), child: ListTile( trailing: Icon(Icons.check_box_outline_blank), title: Text( 'Title ${items[index] + 1}', ), ), background: Container( color: Colors.red, ), onDismissed: (DismissDirection direction) { setState(() { items.removeAt(index); }); }, confirmDismiss: (DismissDirection direction) async { return await showDialog( context: context, builder: (BuildContext context) { return AlertDialog( title: Text("Confirm"), content: Text("Are you sure you wish to delete this item?"), actions: <Widget>[ ElevatedButton( onPressed: () => Navigator.of(context).pop(true), child: Text("Confirm") ), OutlinedButton( onPressed: () => Navigator.of(context).pop(false), child: Text("Cancel"), ), ], ); }, ); }, ); }, ), ); } }