An alert dialog which consists of an optional title and an optional list of actions informs the user about situations that require acknowledgement.
Table of Contents
Open an dialog
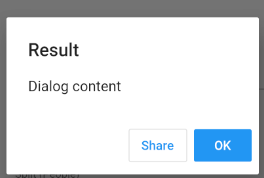
showDialog(
context: context,
barrierDismissible: false,
builder: (_) => AlertDialog(
title: Text('Result'),
content: Text('Dialog content'),
actions: <Widget>[
new OutlineButton(
child: new Text('Share'),
onPressed: () {
},
),
new FlatButton(
child: new Text('OK', style: TextStyle(color: Colors.white),),
color: Colors.blue,
onPressed: () {
Navigator.of(context).pop();
},
),
],
)
);
showDialog() then can be called in any events such as onPressed, onTap.
RaisedButton(onPressed: _calculate)
Prevent dialog from closing from touching outside
barrierDismissible
property determines whether you can dismiss this route by tapping the modal barrier.
showDialog(
context: context,
barrierDismissible: false,
...
)
To close the dialog, call Navigator.of(context).pop()
within onPressed event:
onPressed: () {
Navigator.of(context).pop();
},
Get result from dialog
Dialog
class MyDialog extends StatelessWidget {
final TextEditingController _controller = TextEditingController();
@override
Widget build(BuildContext context) {
return AlertDialog(
title: Text('Add emoji'),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(8.0)),
content: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
TextField(
controller: _controller,
decoration: InputDecoration(labelText: 'Emoji'),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("Cancel")),
FlatButton(
onPressed: () {
Navigator.pop(context, _controller.text);
},
color: Colors.blue,
child: Text("Save", style: TextStyle(color: Colors.white),)),
],
)
],
),
);
}
}
Call dialog and get dialog’s input data in Flutter TextField:
showDialog(
builder: (_) => MyDialog()).then((value)
{
if (value != null){
print(value);
}
});