If you want to clone an array that is identical to the original, then just assigning values one at a time via the assignment operator will not work.
var ages = [20, 25, 45, 54, 62];
var ages2 = ages;
The new variable will have the values from the old one, and it will also refer to the old one. So any changes made to either of them will change in both.
ages.push(18);
console.log(ages); // [20, 25, 45, 54, 62, 18];
console.log(ages2); // [20, 25, 45, 54, 62, 18];
//the change was applied to the cloned array as well
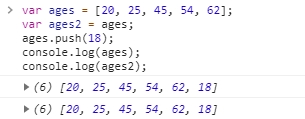
The assignment operator does not copy any values from the original array to the cloned one. It just points the new variable to the original one’s memory location, also known as reference assignment.
To create a new array with its own values that you can change and don’t affect others, you need to make a deep copy of the array. The assignment operator creates a shallow copy.
There are many ways to create a deep copy.
Table of Contents
Destructuring assignment
Deep cloning an array in JavaScript is not that hard. ES6 introduce a simple way to do it is with destructuring assignment syntax. Destructuring operators allow developers to easily break a collection into its own individual pieces. In our scenario, by breaking the items out of the array (and assigning them individually), we can avoid concatenating overloaded values into one variable, and improve scope-related issues greatly.
var randomNumbers = [20, 25, 45, 54, 62];
var [...newNumbers] = randomNumbers;
console.log(newNumbers); //output: [20, 25, 45, 54, 62]
For Loop
We loop through an array and assign each of its children to a new array.
var randomNumbers = [22, 14, 16, 19, 21];
var newNumbers = [];
for(var i = 0; i < randomNumbers.length; i++){
newNumbers.push(randomNumbers[i]);
}
JSON
Both methods mentioned above don’t work with copying an array of objects. Even though we break the array out of the reference, its object children still keep their references.
In this case, we use JSON parse. Thehe JSON.parse() method can take a JSON string and make it into an object. You can supply a function to do something with this object before it gets made, such as changing it or adding something new.
var students = [{age: 20, name: 'John'}, {age: 20, name: 'Billy'}];
var newStudents = JSON.parse(JSON.stringify(students));
newStudents.push({age: 20, name: 'Mary'});
console.log(students);
console.log(newStudents);
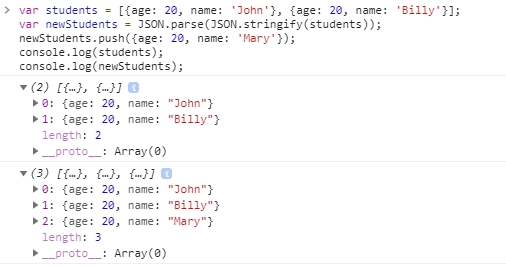
As you see in the log image, both arrays have different elements.