When using JavaScript, if you want to know if an array is empty or not, you can use .length
property,
The length property of an array returns its size. If it is 0, the array is empty.
const languages = []; if(languages.length == 0){ console.log('this array is empty'); } if (!languages .length) { console.log("Array is empty"); } else { console.log("Array is not empty"); }
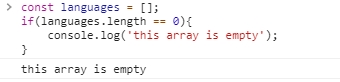
If the length property returns a number bigger than 0, the array is not empty.
const languages = ['Java', 'JavaScript', 'Kotlin', 'Dart']; if(languages.length > 0){ for(var i = 0; i < languages.length; i++) { console.log(languages[i]); } }
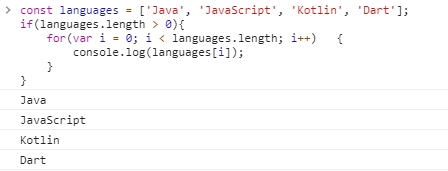
We can loop through the array normally after checking if it is empty or not.
Remember, in JavaScript, an array is considered empty if it doesn’t contain any elements. But it can still contain properties or methods, because in JavaScript, arrays are also objects. If you want to check whether an array-like object has any enumerable properties, you’ll need to use different methods.