If you are trying to figure out which package is best to use with Laravel for user roles and permissions, here are the best two for you.
Table of Contents
Spatie’s Laravel-Permission
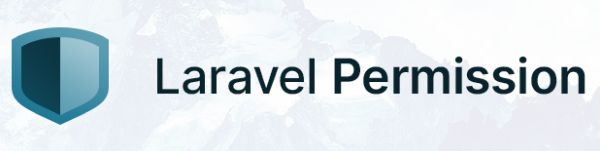
Spatie’s Laravel-Permission library is built on top of Laravel’s authorization features. They were introduced in the 5.1.1 release. This package allows you to manage user permissions and roles in a database and it does the best compared to other packages with the same functionality.
User model
use Illuminate\Foundation\Auth\User as Authenticatable; use Spatie\Permission\Traits\HasRoles; class User extends Authenticatable { use HasRoles; // properties and methods of User class }
Use Laravel-Permisison
// Assign role to user and adding permissions to a role $user->assignRole('webmaster', 'admin'); $role = Role::findByName('webmaster'); $role->givePermissionTo('view stats'); // Adding permissions to a user $user->givePermissionTo('add articles, delete articles, add users, view stats');
Besides allowing the developer to check if a user has a permission or role in PHP code, it also supports Blade directives.
@can('view stats') show status here @endcan
Joseph Silber’s Bouncer
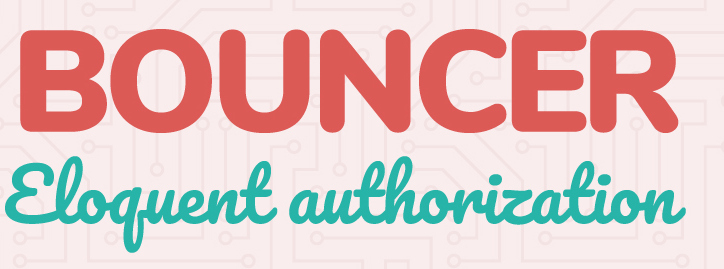
Bouncer is another great approach to managing roles and permission for any app using Eloquent models.
// Adding abilities for users Bouncer::allow($user)->to('ban-articles'); Bouncer::allow($user)->to('edit', Post::class); Bouncer::allow($user)->to('delete', $post); Bouncer::allow($user)->everything(); Bouncer::allow($user)->toManage(Post::class); Bouncer::allow($user)->toManage($post); Bouncer::allow($user)->to('view')->everything(); Bouncer::allow($user)->toOwn(Post::class); Bouncer::allow($user)->toOwnEverything(); // Removing abilities uses the same syntax, e.g. Bouncer::disallow($user)->to('delete', $post); Bouncer::disallow($user)->toManage(Post::class); Bouncer::disallow($user)->toOwn(Post::class); // Adding & removing abilities for roles Bouncer::allow('admin')->to('ban-articles'); Bouncer::disallow('admin')->to('ban-articles'); // You can also forbid specific abilities with the same syntax... Bouncer::forbid($user)->to('delete', $post); // And also remove a forbidden ability with the same syntax... Bouncer::unforbid($user)->to('delete', $post); // Re-syncing a user's abilities Bouncer::sync($user)->abilities($abilities); // Assigning & retracting roles from users Bouncer::assign('admin')->to($user); Bouncer::retract('admin')->from($user); // Assigning roles to multiple users by ID Bouncer::assign('admin')->to([1, 2, 3]); // Re-syncing a user's roles Bouncer::sync($user)->roles($roles); // Checking the current user's abilities $boolean = Bouncer::can('ban-articles'); $boolean = Bouncer::can('edit', Post::class); $boolean = Bouncer::can('delete', $post); $boolean = Bouncer::cannot('ban-articles'); $boolean = Bouncer::cannot('edit', Post::class); $boolean = Bouncer::cannot('delete', $post); // Checking a user's roles $boolean = Bouncer::is($user)->a('subscriber'); $boolean = Bouncer::is($user)->an('admin'); $boolean = Bouncer::is($user)->notA('subscriber'); $boolean = Bouncer::is($user)->notAn('admin'); $boolean = Bouncer::is($user)->a('moderator', 'editor'); $boolean = Bouncer::is($user)->all('moderator', 'editor'); Bouncer::cache(); Bouncer::dontCache(); Bouncer::refresh(); Bouncer::refreshFor($user);
Laravel-ACL
Laravel ACL adds role-based permissions to built-in Auth System of Laravel 6.0+. ACL middleware protects routes and even CRUD controller methods. It requires PHP 7.2+ and Laravel 6.0+ in the latest version.
User model
use Kodeine\Acl\Traits\HasRole; class User extends Model implements AuthenticatableContract, CanResetPasswordContract { use Authenticatable, CanResetPassword, HasRole; }
Usage
//Create a new role $roleAdmin = new Role(); $roleAdmin->name = 'Administrator'; $roleAdmin->slug = 'administrator'; $roleAdmin->description = 'manage administration privileges'; $roleAdmin->save(); //create a permission $permission = new Permission(); $permPost = $permission->create([ 'name' => 'post', 'slug' => [ // pass an array of permissions. 'create' => true, 'view' => true, 'update' => true, 'delete' => true, ], 'description' => 'manage post' ]); //assign permission to role $roleAdmin = Role::first(); // administrator // permission as an object $roleAdmin->assignPermission($permUser); // as an id $roleAdmin->assignPermission($permUser->id); // or by name $roleAdmin->assignPermission('user'); // or by collection $roleAdmin->assignPermission(Permission::all());