WordPress plugin boilerplate is a set of files and code that provides a foundation for developers to quickly create a WordPress plugin. It is designed to be a starting point for developers to build their custom plugins. It includes all the necessary files and code to get a plugin up and running, as well as a comprehensive set of guidelines and best practices to ensure the plugin is secure and efficient.
The following is a collection of boilerplate for quickly building WordPress plugins.
Table of Contents
WordPress plugin structure
This part contains the basic file structure for placing WordPress plugin files. There is no working code in this compressed file.
This structure is used to upload the plugin to WordPress’s plugin repository.
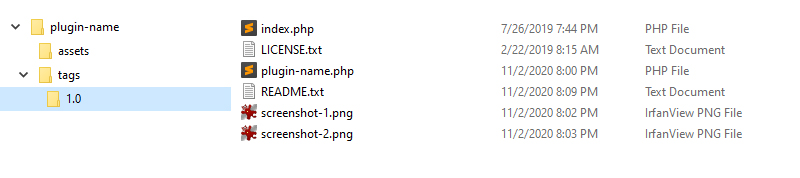
If you want to use this plugin for a WordPress app, just rename 1.0
to plugin-name
and copy it to wp-content\plugins
.
Features
Shortcode
class ShortcodeName { //allow script when the shortcode exists in a post static $hasShortcode; static function init() { add_shortcode('shortcode_name', array(__CLASS__, 'shortcode_function')); add_action('init', array(__CLASS__, 'register_scripts')); add_action('wp_footer', array(__CLASS__, 'print_scripts')); add_action('wp_head', array(__CLASS__, 'print_styles')); } static function shortcode_function($atts) { self::$hasShortcode = true; extract(shortcode_atts(array( 'att1' => 'Hello', 'att2' => 1000, ), $atts)); $output = "$att1 and $att2"; return $output; } //register scripts and styles static function register_scripts() { wp_register_script('script-name', plugins_url('script-name.js', __FILE__), array('jquery'), '1.0', true); wp_register_style( 'style-name', plugins_url( 'style-name.css', __FILE__ ), true, '', 'all' ); } //print scripts if shortcode_name exists static function print_scripts() { if (!self::$hasShortcode) return; wp_print_scripts('script-name'); } //print styles if shortcode_name exists static function print_styles() { if (!self::$hasShortcode) return; wp_print_styles( 'style-name' ); } } ShortcodeName::init();
Admin Page
function plugin_name_admin_menu() { add_menu_page( 'Top Level', 'Top Level Menu', 'manage_options', 'plugin-name-admin-page', 'plugin_name_admin_page', 'dashicons-tickets', 10 ); add_submenu_page( 'plugin-name-admin-page', 'My Sub Level Menu Example', 'Sub Level Menu', 'manage_options', 'plugin-name-admin-sub-page', 'plugin_name_admin_sub_page' ); } add_action( 'admin_menu', 'plugin_name_admin_menu' ); function plugin_name_admin_page(){ $output = '<div class="wrap"> <h1 class="wp-heading-inline">Title</h1> <a href="#" class="page-title-action">Add New</a> <hr class="wp-header-end"> <div id="poststuff"> <div class="postbox"> <h3 class="hndle"> <span>Box title</span> </h3> <div class="inside"> Content </div> </div> </div> </div>'; _e($output); } function plugin_name_admin_sub_page(){ $output = '<div class="wrap"> <h1 class="wp-heading-inline">Title</h1> <a href="#" class="page-title-action">Add New</a> <hr class="wp-header-end"> <div id="poststuff"> <div class="postbox"> <h3 class="hndle"> <span>Box title</span> </h3> <div class="inside"> Content </div> </div> </div> </div>'; _e($output); }