With the support of WebRTC, real-time communication capabilities in an app browser become reality. It supports many types of data, including voice, which allows developers to build powerful voice commands, text-to-speech, and speech recognition solutions.
Table of Contents
SpeechSynthesis
The SpeechSynthesis interface of the Web Speech API is the controller interface for the speech service.
var msg = new SpeechSynthesisUtterance(); msg.text = "This is my message. Hello world."; window.speechSynthesis.speak(msg);
You can test these 3 lines of code in Developer Tools.
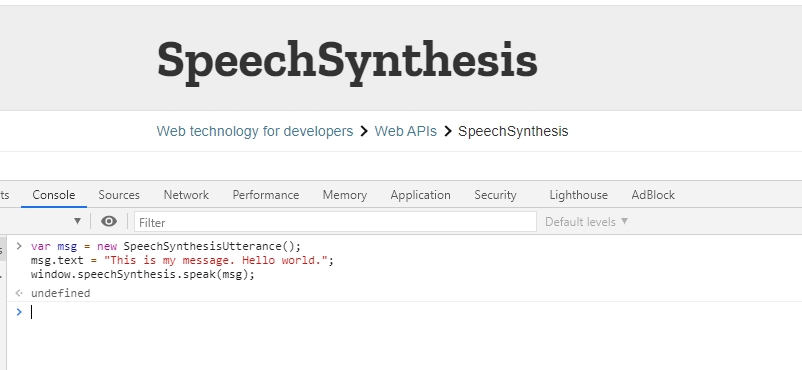
voice-commands.js
voice-commands.js is a simple wrapper for Javascript Speech-to-text to add voice commands.
f ( SPEECH.isCapable() ) { // the browser supports speech recognition SPEECH.onStart(function() { // fires once browser recognition has started }); SPEECH.onStop(function() { // fires when speech is manually stopped, or on error }); SPEECH.min_confidence = .2; // the default minimum confidence you're willing to accept as a command SPEECH.addVoiceCommands([ { command: "show help", callback: function() { // do something when the user says "show help". Maybe open a help dialog! }, min_confidence: .5 // you can set a confidence level for each command individually }, { command: /next (slide)?/, callback: function() { // this would fire when the user says "next" OR "next slide" // using a regex like that makes the voice command recognition // a bit more forgiving } }, { command: /go.+(top|home)/, // regex to match commands more dynamically callback: function() { // the regex above would match: // * go home // * go to the top } } }); SPEECH.onResult(function(result) { // fires after commands set via addVoiceCommands are parsed. // result.transcript is the object built by the speech recognition engine. // result.confidence is confidence in decimals (0.02392) }); // gets things going. when speech recognition is ready, // onStart will be called. // you can also pass config here SPEECH.start({ min_confidence: .3, lang: 'en-US' // defaults to HTML lang attribute value, or user agent's language }); }
juliusjs
Julius is a high-performance, small-footprint large vocabulary continuous speech recognition (LVCSR) decoder software for speech-related researchers and developers. JuliusJS is its JavaScript port. The library actively listens to the user to transcribe what they are saying through a callback.
var julius = new Julius(); julius.onrecognition = function(sentence) { console.log(sentence); };
Text To Speech JS
This is a small JavaScript library that provides a text-to-speech conversion using tts-api.com service.
TextToSpeech.talk("Hello TL Dev Tech!");
Pocketsphinx.js
Pocketsphinx.js is a speech recognition library, ported from PocketSphinx. So Pocketsphinx.js’ features are tightly related to the features of PocketSphinx.
- All-JavaScript API,
- Calls can be made through Web Workers or not,
- Supports all acoustic models supported by PocketSphinx,
- Supports most of the command-line parameters of PocketSphinx,
- Support for Finite State Grammars (FSG) input from JavaScript,
- Support for Statistical Language Models or JSGF grammars input from files,
- Support for Keyword spotting,
- Optional audio recording library for real-time recognition.
script type="text/javascript"> var Module = { locateFile: function() {return "/path/to/pocketsphinx.wasm";}, onRuntimeInitialized: function() { // Now I can start using it ... } }; </script> <script src="path/to/pocketsphinx.js"></script>
Artyom.js
Artyom.js is a useful wrapper of the speechSynthesis
and webkitSpeechRecognition
APIs. Besides, artyom.js also lets you to add voice commands to your website easily.
// If you are using VanillaJS const artyom = new Artyom(); var commandHello = { indexes:["hello","good morning","hey"], // These spoken words will trigger the execution of the command action:function(){ // Action to be executed when a index match with spoken word artyom.say("Hey buddy ! How are you today?"); } }; artyom.addCommands(commandHello);
Annyang
annyang is a tiny JavaScript library that lets your visitors control your site with voice commands. annyang supports multiple languages, has no dependencies, weighs just 2kb, and is free to use.
if (annyang) { // Let's define our first command. First the text we expect, and then the function it should call var commands = { 'show tps report': function() { $('#tpsreport').animate({bottom: '-100px'}); } }; // Add our commands to annyang annyang.addCommands(commands); // Start listening. You can call this here, or attach this call to an event, button, etc. annyang.start(); }
Voix.js
voix.js allows developers to add voice commands to their sites, apps or games.
var voix = new Voix('en-US'); voix.setCommand('play', playVideo);