Data tables are an important part of any admin dashboard. It helps show data to webmasters and users. Creating a data table to enhance a website can be made simpler with the help of jQuery and JavaScript libraries.
These libraries offer a wide range of features, such as the ability to create dynamic tables, sort and filter data, etc. This article will discuss 6 of the best jQuery and JavaScript data table libraries that can help you create an effective data table for your website.
Table of Contents
DataTables
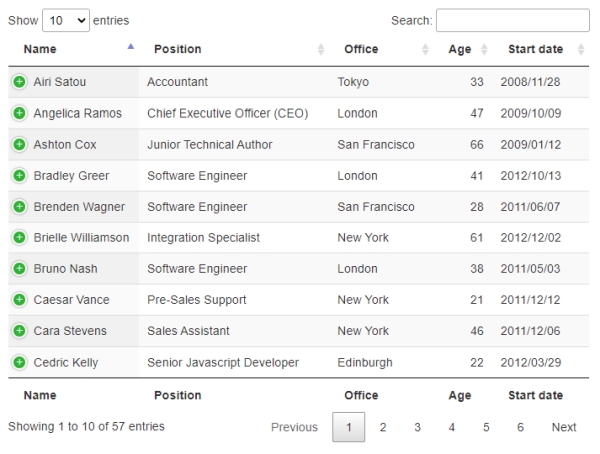
This is the most popular data table library for jQuery and JavaScript. It supports pagination, instant search, multi-column ordering, remote data source, themes, and extensions.
$(document).ready( function () { $('table').DataTable(); } );
Clusterize.js
This is a plugin for any tables with a large dataset. It can work smoothly with over 400,000 rows.
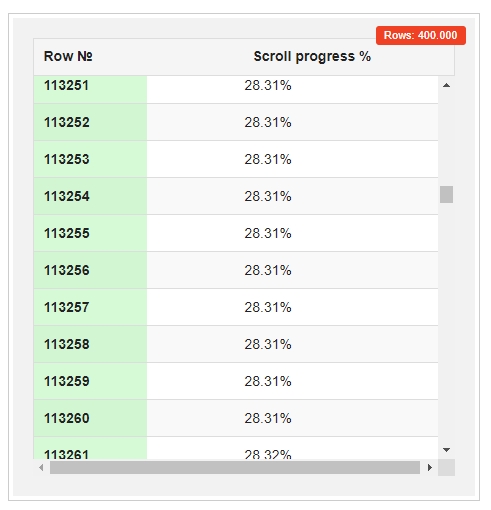
var data = ['<tr>…</tr>', '<tr>…</tr>', …]; var clusterize = new Clusterize({ rows: data, scrollId: 'scrollArea', contentId: 'contentArea' });
Handsontable
Handsontable is a JavaScript library that makes a data grid feel like a spreadsheet. It is very easy to implement, flexible, and super customizable. You can extend it with custom plugins and edit the source code to adjust it to your product.
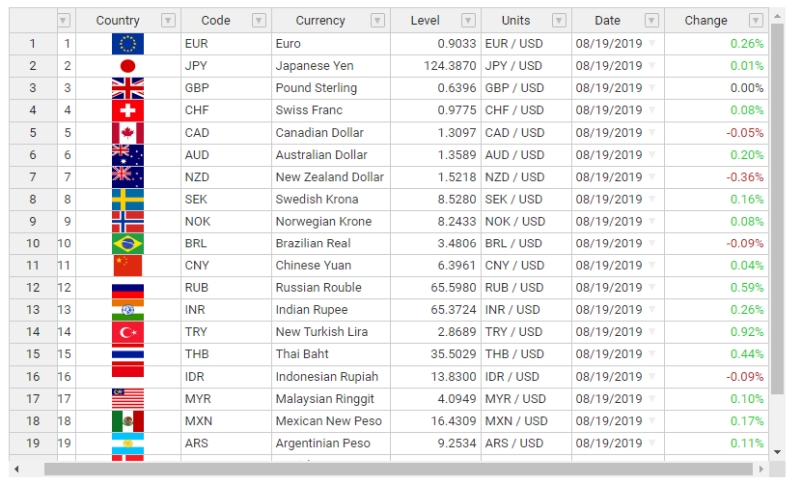
jTable
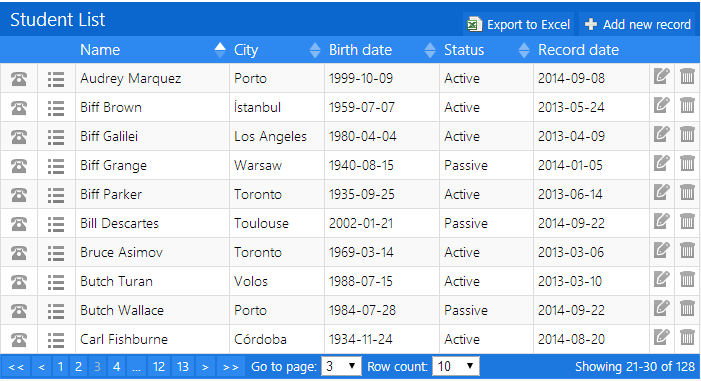
jTable is a jQuery plugin used to create AJAX-based CRUD tables. The plugin has many useful features, including AJAX data source, ‘create new record’ with jQueryUI dialog form, server-side paging using AJAX, server-side sorting using AJAX, row selection, and many more.
$(document).ready(function () { $('#StudentTableContainer').jtable({ title: 'The Student List', paging: true, //Enable paging pageSize: 10, //Set page size (default: 10) sorting: true, //Enable sorting defaultSorting: 'Name ASC', //Set default sorting actions: { listAction: '/Demo/StudentList', deleteAction: '/Demo/DeleteStudent', updateAction: '/Demo/UpdateStudent', createAction: '/Demo/CreateStudent' }, fields: { StudentId: { key: true, create: false, edit: false, list: false }, Name: { title: 'Name', width: '23%' }, EmailAddress: { title: 'Email address', list: false }, Password: { title: 'User Password', type: 'password', list: false }, Gender: { title: 'Gender', width: '13%', options: { 'M': 'Male', 'F': 'Female' } }, CityId: { title: 'City', width: '12%', options: '/Demo/GetCityOptions' }, BirthDate: { title: 'Birth date', width: '15%', type: 'date', displayFormat: 'yy-mm-dd' }, Education: { title: 'Education', list: false, type: 'radiobutton', options: { '1': 'Primary school', '2': 'High school', '3': 'University' } }, About: { title: 'About this person', type: 'textarea', list: false }, IsActive: { title: 'Status', width: '12%', type: 'checkbox', values: { 'false': 'Passive', 'true': 'Active' }, defaultValue: 'true' }, RecordDate: { title: 'Record date', width: '15%', type: 'date', displayFormat: 'dd.mm.yy', create: false, edit: false, sorting: false //This column is not sortable! } } }); //Load student list from server $('#StudentTableContainer').jtable('load'); });
Tabulator
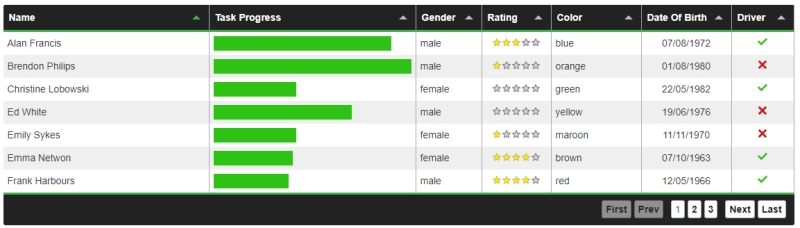
Tabular has all the features which are included in DataTables library.
var table = new Tabulator("#example-table", { data:tabledata, //load row data from array layout:"fitColumns", //fit columns to width of table responsiveLayout:"hide", //hide columns that dont fit on the table tooltips:true, //show tool tips on cells addRowPos:"top", //when adding a new row, add it to the top of the table history:true, //allow undo and redo actions on the table pagination:"local", //paginate the data paginationSize:7, //allow 7 rows per page of data movableColumns:true, //allow column order to be changed resizableRows:true, //allow row order to be changed initialSort:[ //set the initial sort order of the data {column:"name", dir:"asc"}, ], columns:[ //define the table columns {title:"Name", field:"name", editor:"input"}, {title:"Task Progress", field:"progress", hozAlign:"left", formatter:"progress", editor:true}, {title:"Gender", field:"gender", width:95, editor:"select", editorParams:{values:["male", "female"]}}, {title:"Rating", field:"rating", formatter:"star", hozAlign:"center", width:100, editor:true}, {title:"Color", field:"col", width:130, editor:"input"}, {title:"Date Of Birth", field:"dob", width:130, sorter:"date", hozAlign:"center"}, {title:"Driver", field:"car", width:90, hozAlign:"center", formatter:"tickCross", sorter:"boolean", editor:true}, ], });
Cheetah Grid
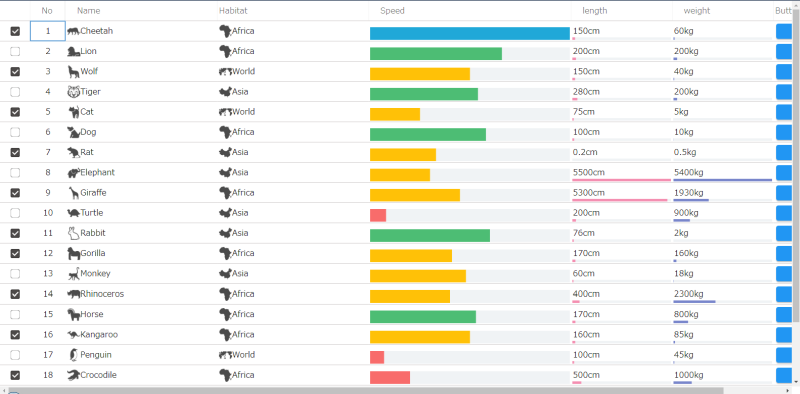
Cheetah Grid is among the frequently updated data table plugins. It allows users not only to convert the current table to a dynamic one with searching, and pagination but also to modify data from the script.
<div id="sample" style="height: 300px; border: solid 1px #ddd;"> </div> <script> // initialize const grid = new cheetahGrid.ListGrid({ // Parent element on which to place the grid parentElement: document.querySelector('#sample'), // Header definition header: [ {field: 'check', caption: '', width: 50, columnType: 'check', action: 'check'}, {field: 'personid', caption: 'ID', width: 100}, {field: 'fname', caption: 'First Name', width: 200}, {field: 'lname', caption: 'Last Name', width: 200}, {field: 'email', caption: 'Email', width: 250}, ], // Array data to be displayed on the grid records, // Column fixed position frozenColCount: 2, }); </script>