Dart language is a client-optimized language for fast apps on any platform, made by Google. It is a programming language optimized for developing user interfaces.
Table of Contents
Flutter
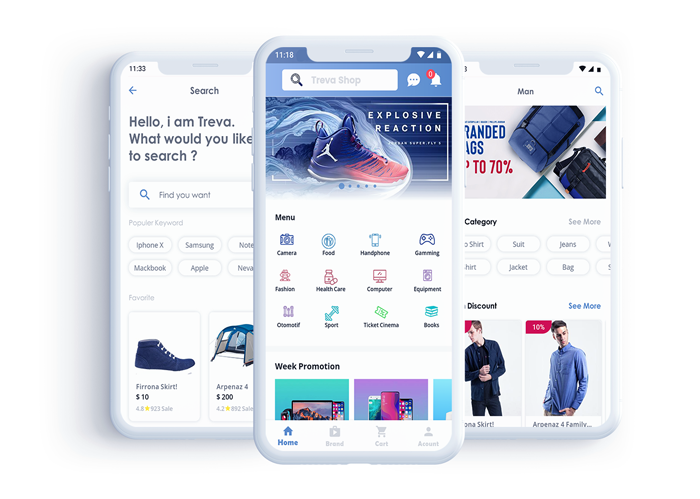
Flutter is an open-source framework that can be used to build native-looking Android and iOS apps, and web, and desktop apps (Windows, Linux, and Mac) with the same code base.
Features:
- One code base – Flutter doesn’t need any platform-specific UI components to render its UI. You only need to write one logic and UI then it can be built to any specific platform the framework supports.
- Hot Reload – Flutter features Hot Reload which allows developers to see the applied changes almost instantly, without even losing the current app’s state. In the long run, it saves time, money, and effort.
- Native performance – Depending on the OS a Flutter app runs on, you get access to widgets that are based on the platform differences of Android and iOS, Windows, Linux, and Mac. Furthermore, the Flutter app is built directly into the machine code.
- Complex UI and Animation made easy – If you used to write a list (a single component), you will know how much boilerplate it requires just to make a simple list. That doesn’t even count more complicated UI stacks. And animation is another beast developer needs to tame. Both of them can be done easier in Flutter.
- Own rendering engine – Flutter uses Skia for rendering. Thanks to the engine, UI built-in Flutter can be launched on virtually any platform.
- Large widget library – Despite being new, Flutter’s built-in widget collection is huge and can be used in almost any situation. Furthermore, 3rd-party libraries are increasing day by day.
Conduit
Conduct is an open-source web framework that enables developers to create web and server applications. It provides an extensive range of features, such as a Dart HTTP server framework, routing, a statically-typed ORM, a database migration option similar to Django, an OAuth 2.0 server and security model, and an integrated testing library. This framework is free to use and provides all the necessary tools to develop enterprise-level apps.
final delegate = ManagedAuthDelegate<User>(context); authServer = AuthServer(delegate); router.route("/profile") .link(() => Authorizer.bearer( authServer, scopes: ["profile.readonly"])) .link(() => ProfileController(context));
Start
Start is a web development framework for Dart, inspired by the Sinatra framework. It offers custom responses and requests, advanced routes, web socket routes, and other helpful features.
It is a great choice for creating REST-API server apps but does not include an ORM module, Auth library, or template engine.
import 'package:start/start.dart'; void main() { start(port: 3000).then((Server app) { app.static('web'); app.get('/hello/:name.:lastname?').listen((request) { request.response .header('Content-Type', 'text/html; charset=UTF-8') .send('Hello, ${request.param('name')} ${request.param('lastname')}'); }); app.ws('/socket').listen((socket) { socket.on('ping').listen((data) => socket.send('pong')); socket.on('pong').listen((data) => socket.close(1000, 'requested')); }); }); }
Aqueduct
Aqueduct is an extensible HTTP framework for building REST APIs on top of the Dart VM. It includes a statically-typed ORM, OAuth 2.0 provider, automated testing libraries, and OpenAPI 3.0 integration.
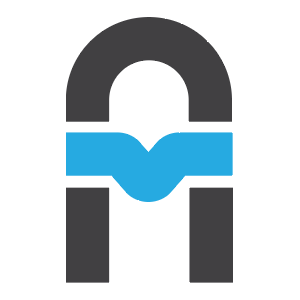
Features:
- Multi-threaded – Developers don’t need to write separate code for leveraging every CPU. Aqueduct’s memory-isolated threads already do that our of the box.
- Fluid Routing – Routes are defined with higher-order functional syntax, which makes application flow easy to construct and read.
- Statically-typed ORM and Database Migration – The built-in ORM makes database query a breeze with powerful, statically typed queries. It stores data in database tables and maps table rows to Dart objects.
- OAuth 2.0 Server – OAuth 2.0 server can be created in just a few lines of code. Tokens and client identifiers are supported via a layered implementation.
- Productive Request Bindings – The bindings approach help to avoid simple errors and make sure your API handles errors correctly and consistently.
- Integrated Test Library – Any Aqueduct app can be run, tested, debugged, and profiled. You can integrate continuous integration tools like TravisCI with ease.
- OpenAPI 3 Integration – Developers can generate OpenAPI 3 documents from your source code and deploy first-class documentation automatically.
Angle
Angel is a complete and production-ready backend framework written in Dart. It provides the tools necessary to create powerful and efficient web applications with features such as full client-side support, GraphQL integration, an authentication library, seamless application configuration, and a strong validation library. It also features ORM support for both PostgreSQL and MongoDB databases and a highly capable templating engine.
import 'package:angel_framework/angel_framework.dart'; import 'package:angel_framework/http.dart'; main() async { var app = Angel(), http = AngelHttp(); app ..get('/', (req, res) => res.writeln('Hello, Angel!')); ..fallback((req, res) => throw AngelHttpException.notFound()); await http.startServer('127.0.0.1', 3000); print('Listening at ${http.uri}'); }