ClipPath in Flutter is a widget that clips its child using a path. We use ClipPath to make the child widget appear masked in our designed shape.
If you want to animate ClipPath, read this post.
Table of Contents
Wave
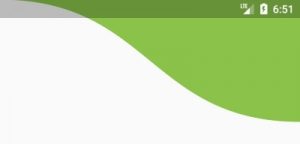
ClipPath( clipper: WaveShape(), child: Container( width: MediaQuery.of(context).size.width, height: 160, color: Colors.lightGreen, ), )
class WaveShape extends CustomClipper<Path> { @override getClip(Size size) { double height = size.height; double width = size.width; var p = Path(); p.lineTo(0, 0); p.cubicTo(width * 1 / 2, 0, width * 2 / 4, height, width, height); p.lineTo(width, 0); p.close(); return p; } @override bool shouldReclip(CustomClipper oldClipper) => true; }
Wave
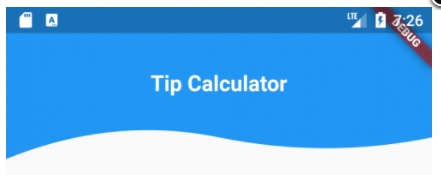
ClipPath( clipper: WaveClip(), child: Container( color: Colors.blue, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ title, ], )
class WaveClip extends CustomClipper<Path> { @override Path getClip(Size size) { Path path = new Path(); final lowPoint = size.height - 20; final highPoint = size.height - 40; path.lineTo(0, size.height); path.quadraticBezierTo( size.width / 4, highPoint, size.width / 2, lowPoint); path.quadraticBezierTo( 3 / 4 * size.width, size.height, size.width, lowPoint); path.lineTo(size.width, 0); return path; } @override bool shouldReclip(CustomClipper<Path> oldClipper) { return false; } }
Round bottom
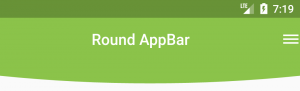
ClipPath( clipper: RoundShape(), child: Container( height: 40, color: Colors.lightGreen, ), )
class RoundShape extends CustomClipper<Path> { @override getClip(Size size) { double height = size.height; double width = size.width; double curveHeight = size.height / 2; var p = Path(); p.lineTo(0, height - curveHeight); p.quadraticBezierTo(width / 2, height, width, height - curveHeight); p.lineTo(width, 0); p.close(); return p; } @override bool shouldReclip(CustomClipper oldClipper) => true; }