Have you ever tried to animate the opacity of a Flutter widget? It’s actually super easy with AnimatedOpacity widget.
AnimatedOpacity is an animated version of Opacity widget. The widget is a great way to keep your child’s opacity level animation consistent over time, without having to painstakingly adjust it manually.
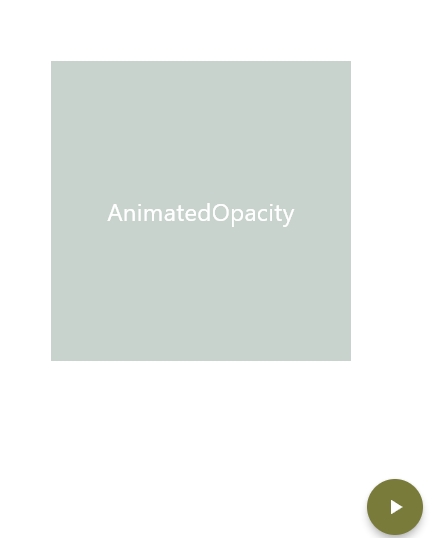
Here is an example of how to use the Flutter AnimatedOpacity widget. This widget allows you to animate opacity from 0% (invisible) to 100% (fully visible).
AnimatedOpacity has 3 required properties which are child, duration and opacity. To make the widget animate its opacity value, we need to set one initial value and other value to transition to.
I used FlexColorScheme to mage the app’s theme and color in this example.
import 'package:flex_color_scheme/flex_color_scheme.dart'; import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> { bool _blur = false; @override Widget build(BuildContext context) { return Scaffold( body: Center( child: AnimatedOpacity( opacity: _blur ? 0.25 : 1, duration: Duration(seconds: 1), child: Container( color: FlexColorScheme.light(scheme: FlexScheme.money).primary, width: 300, height: 300, child: Center( child: Text( 'AnimatedOpacity', style: TextStyle(fontSize: 24.0, color: Colors.white), )), ), ), ), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { _blur = !_blur; }); }, child: Icon(Icons.play_arrow), ), ); } }