AnimatedModalBarrier widget prevents users to interact with its child, which is a widget behind it.
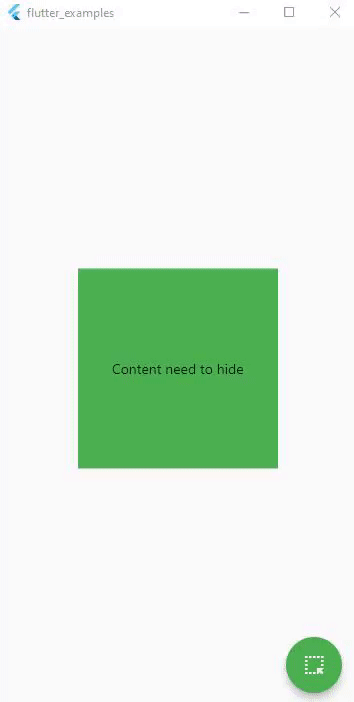
In this AnimatedModalBarrier example, we try showing a barrier that hide the content.
import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> with SingleTickerProviderStateMixin{ late AnimationController _controller; late Animation<Color?> _color; @override void initState() { ColorTween? _colorTween = ColorTween( begin: null, end: Colors.blue.withOpacity(0.25), ); _controller = AnimationController( vsync: this, duration: const Duration(seconds: 1) ); _color = _colorTween.animate(_controller); super.initState(); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Stack( children: [ Align( alignment: Alignment.center, child: Container( width: 200, height: 200, color: Colors.green, child: Center(child: Text('Content need to hide')), ), ), AnimatedModalBarrier(color: _color) ], ), ), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { if(_controller.status == AnimationStatus.completed){ _controller.reverse(); } else { _controller.forward(); } }); }, backgroundColor: Colors.green, child: Icon(Icons.highlight_alt_sharp), ), ); } }