In this article, we will try creating an AppBar with round bottom.
Table of Contents
Use AppBar’s shape
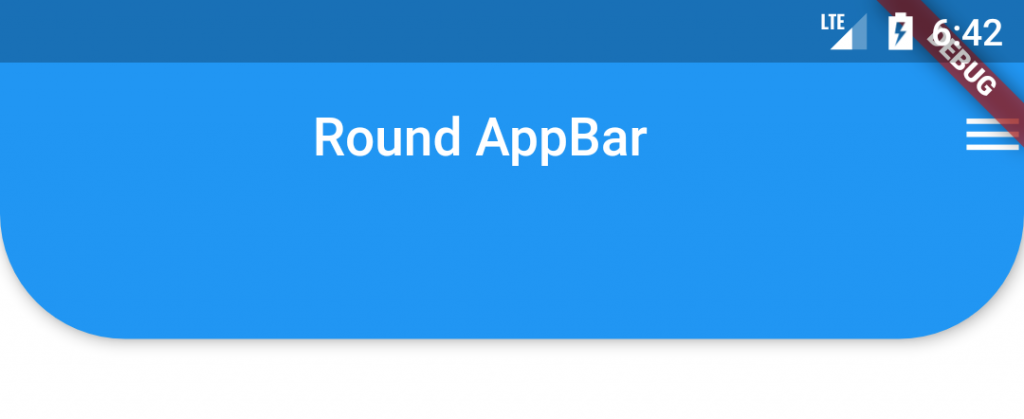
If you want to adjust the height of the AppBar, just modify the barHeight
property. kToolbarHeight
is the height of the toolbar component of the AppBar, which is a constant with the value of 56.0.
Firstly, create a widget which define the appbar.
class RoundAppBar extends StatelessWidget with PreferredSizeWidget{ final double barHeight = 50; final String title; RoundAppBar({Key key, this.title}) : super(key: key); @override Size get preferredSize => Size.fromHeight(kToolbarHeight + barHeight); @override Widget build(BuildContext context) { return AppBar( title: Center(child: Text(title)), shape: RoundedRectangleBorder( borderRadius: BorderRadius.vertical( bottom: Radius.circular(48.0), ), ), actions: [ Icon(Icons.dehaze) ], ); } }
We can include it in a Scaffold like this:
Scaffold( appBar: RoundAppBar(title: 'Round AppBar',), backgroundColor: Colors.white, body: SafeArea( child: Center(child: Text('Body'),) ), );
Use ClipPath
To use ClipPath, we need 2 parts. The first one is a normal rectangle AppBar and a ClipPath under it with the same color.
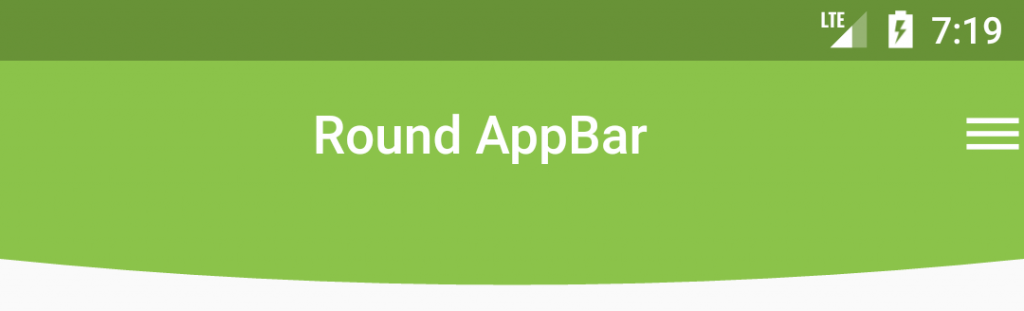
class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Center(child: Text('Round AppBar')), backgroundColor: Colors.lightGreen, elevation: 0, actions: [Icon(Icons.dehaze_sharp)], ), body: SafeArea( child: Column( children: [ ClipPath( clipper: RoundShape(), child: Container( height: 40, color: Colors.lightGreen, ), ), Center( child: Text('Body'), ), ], )), ); } } class RoundShape extends CustomClipper<Path> { @override getClip(Size size) { double height = size.height; double width = size.width; double curveHeight = size.height / 2; var p = Path(); p.lineTo(0, height - curveHeight); p.quadraticBezierTo(width / 2, height, width, height - curveHeight); p.lineTo(width, 0); p.close(); return p; } @override bool shouldReclip(CustomClipper oldClipper) => true; }