It is easier to create a Youtube embed video by using the pre-made code supplied by Youtube. Under a video, you click on “Share” then “Embed”. There will be an iframe code that can be pasted into any sites which support HTML.
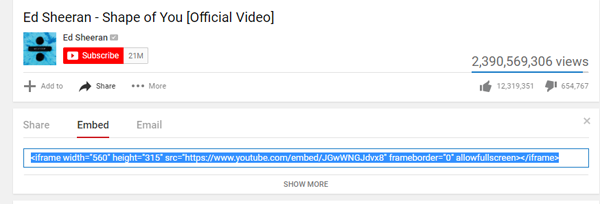
However, this post wants to introduce a way for those, mostly developers, who want to display embedded videos only after a user clicks on a link or any button. Furthermore, we want to make the video player responsive as well.
Table of Contents
HTML
We will create a link for the click event and a wrapper to display the video player.
<a href="https://www.youtube.com/watch?v=JGwWNGJdvx8" class="video-link">Open this video</a> <div id="video-player"></div>
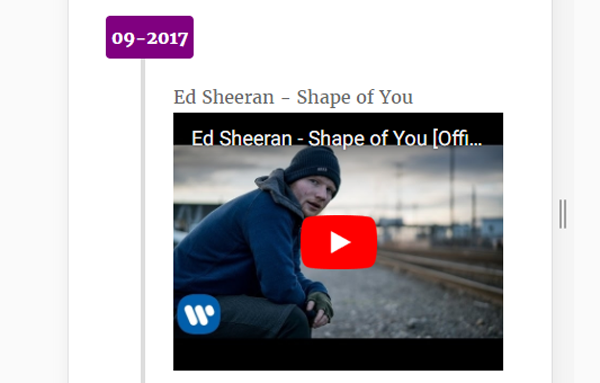
Javascript
jQuery(document).ready(function(){ jQuery('.video-link').on('click', function(e){ var url = jQuery(this).attr('href'); var video_id = new RegExp('[\\?&amp;]v=([^&amp;#]*)').exec(url); var embed_url = ''; if (video_id &amp;&amp; video_id[1]) { embed_url = 'http://youtube.com/embed/' + video_id[1]; } var embed_code = '&lt;iframe width="560" height="315" src="' + embed_url + '" frameborder="0" allowfullscreen&gt;&lt;/iframe&gt;'; jQuery('#video-player').addClass('video-player-opened').html(embed_code); e.preventDefault(); }); });
The reason I add the “video-player-opened” class is to apply a responsive style to only opened video player so it doesn’t mess up its parent’s style.
Style
The embed code isn’t responsive so we need to make it scale depending on a device’s resolution.
.video-player-opened{ position:relative; padding-bottom:56.25%; height:0; overflow:hidden; } .video-player-opened iframe{ position:absolute; top:0; left:0; width:100%; height:100%; }
Why padding-bottom:56.25%;? This number is calculated by using the video’s aspect ratio of 16*9 (9 / 16 = 0.5625).