In this tutorial, we will be discussing how to find the longest word in a string using JavaScript. You may think that you don’t need to know this for your job or maybe you’re not even a programmer. But if you can use this technique, then it could be very beneficial to know how it works! So just go along with me and see what we can do together.
Some methods which will be used in this tutorial:
- split() – This method is used to break a string into words. The delimeter here is blank space. After breaking into words, we can collect all of their lengths and use them to compare.
- map() – The map() method is used to create a new array by mapping each element from the calling array and calling it with an internal function. We use this method to map all separated words’ lengths into an array.
- Math.max – The Math.max() function retrieves the largest of any number of numbers given as parameters, or NaN if an input is not numeric and cannot be converted into a number. This function is used to get the longest length in the array returned by map().
- Spread syntax (…) – Spread syntax, also known as the spread operator, allows an iterable object to be expanded.
Find the Longest Words
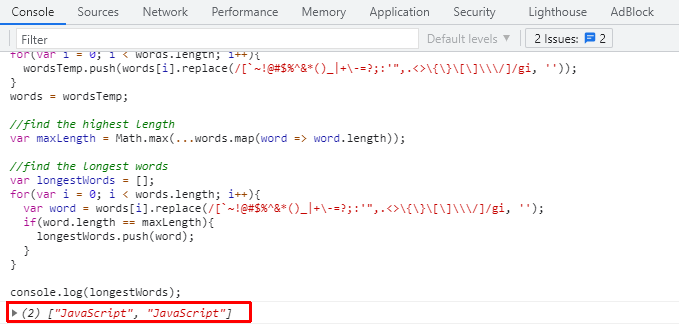
const source = "JavaScript Arrays are a special type of variable that stores a list of values. In JavaScript, an array is written as [item1, item2 ...] and can be either numeric or string literals."; var words = source.split(" "); //remove special characters var wordsTemp = []; for(var i = 0; i < words.length; i++){ wordsTemp.push(words[i].replace(/[`~!@#$%^&*()_|+\-=?;:'",.<>\{\}\[\]\\\/]/gi, '')); } words = wordsTemp; //find the highest length var maxLength = Math.max(...words.map(word => word.length)); //find the longest words var longestWords = []; for(var i = 0; i < words.length; i++){ var word = words[i].replace(/[`~!@#$%^&*()_|+\-=?;:'",.<>\{\}\[\]\\\/]/gi, ''); if(word.length == maxLength){ longestWords.push(word); } } console.log(longestWords);