Before using an object’s property in JavaScript, we must first check if it exists. Otherwise, an error or warning will be thrown instead.
There are 2 ways to detect whether a specified property exists in a specific object.
Table of Contents
Use in
operator
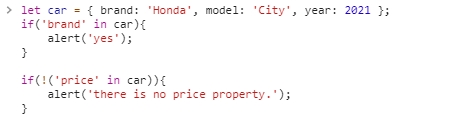
in
operator returns true
if an object or its prototype chain contains the specified property.
let car = { brand: 'Honda', model: 'City', year: 2021 }; if('brand' in car){ alert('yes'); } if(!('price' in car)){ alert('there is no price property.'); } const person = { name: 'John', age: 30, city: 'New York' }; if ('age' in person) { console.log('person object has age property'); } else { console.log('person object does not have age property'); }
Use hasOwnProperty method
This object.hasOwnProperty()
can be used to check an object’s own keys and will only return true
if key
is available on object
directly.
let car = { brand: 'Honda', model: 'City', year: 2021 }; if(car.hasOwnProperty('brand')){ alert('brand exists'); } const person = { name: 'John', age: 30, city: 'New York' }; if (person.hasOwnProperty('age')) { console.log('person object has age property'); } else { console.log('person object does not have age property'); }
Both of these methods will return true
if the object has the specified property and false
if it does not. It’s important to note that if you use the in
operator, it will also return true
if the property exists in the object’s prototype chain. The hasOwnProperty()
method, on the other hand, only checks if the property exists directly on the object itself.