JavaScript Array is a data type that stores ordered sets of values. The JavaScript language has several built-in methods for working with arrays, including push(), pop(), and reverse(). These methods are often used to manipulate lists in the document object model (DOM) or within loops. Arrays can be initialized using an array literal or by calling new Array() with a list of items as arguments. Similarly, they can be created from existing objects via the Object.create() method’s array parameter or by passing one argument after another while setting their properties individually.
Inserting an item into an array at a specific index is a fairly common programming task. It might seem like this should be something that can easily be done, but it’s not so easy if you don’t what JavaScript offers. In this article we will show you how to insert an item into an array at any index using JavaScript and also provide some examples of when you might need to do such a thing.
In JavaScript, there is splice() method which can modify content of an array.
The splice() method modify an array by removing an item or adding a new item in specific index.
Table of Contents
Insert an item
This example shows how to insert an object after the 2nd item of an array.
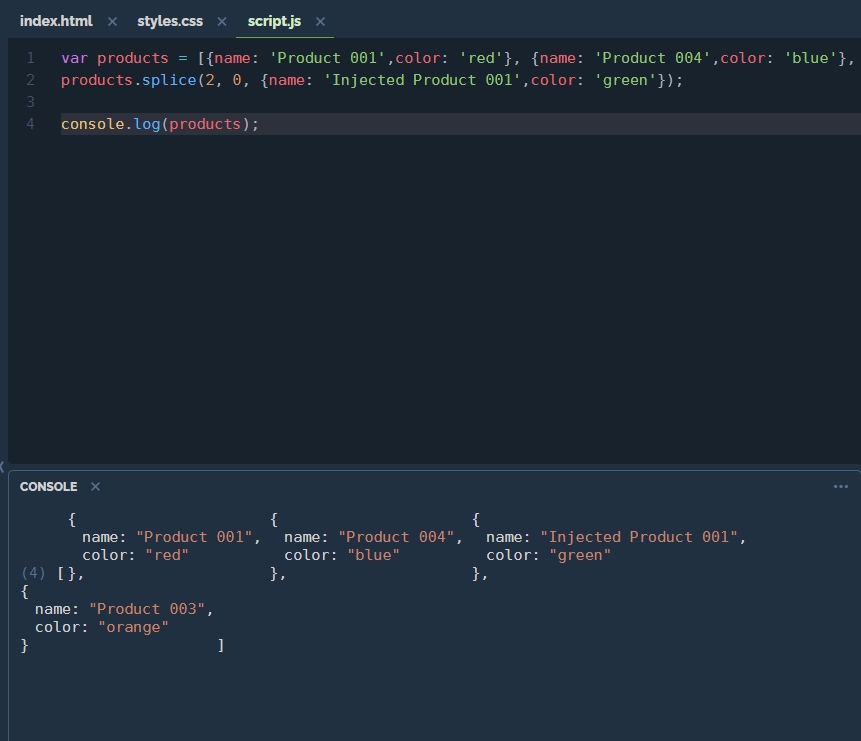
var products = [{name: 'Product 001',color: 'red'}, {name: 'Product 004',color: 'blue'}, {name: 'Product 003',color: 'orange'}]; products.splice(2, 0, {name: 'Injected Product 001',color: 'red'}); console.log(products); //output: Array [Object { name: "Product 001", color: "red" }, Object { name: "Product 004", color: "blue" }, Object { name: "Injected Product 001", color: "red" }, Object { name: "Product 003", color: "orange" }]
Turn it into a method
Array.prototype.insertAt = function ( index, item ) { this.splice( index, 0, item ); }; //call it products.insertAt(2, {name: 'Injected Product 001',color: 'red'});
We hope that this article has helped you understand how to do so, and also when you might need to insert items in arrays using JavaScript. In the future if you are faced with such a situation be sure to refer back here for some tips! Have any questions or comments? Let us know below.