JavaScript arrays are a powerful and versatile data type that can be used to store any type of information. One way to use an array is by using it as a list of items. There may be moments when you need to remove something from the array, such as a specific item or all the items in the array. This blog post will guide you how to do this with examples for both removing one item and removing all items in an array.
Table of Contents
Use splice()
The splice() function can be used to change the contents of an array by removing, replacing, or adding new elements at any given location.
var numbers = [10, 16, 17, 25, 26, 31, 36, 37, 39, 42];
var index = numbers.indexOf(25);
if (index > -1) {
numbers.splice(index, 1);
}
console.log(numbers);
//output: [10, 16, 17, 26, 31, 36, 37, 39, 42]
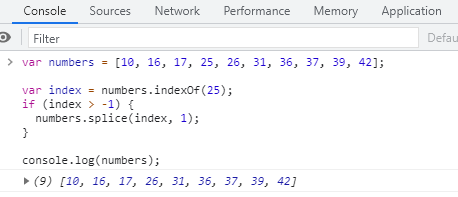
Use filter()
The filter() method creates a new array with all items that pass the specified test.
var languages = ["Java", "Kotlin", "Swift", "C#"];
var needle = "Swift";
languages = languages.filter(function(language) {
return language !== needle;
});
console.log(languages);
//output: ["Java", "Kotlin", "C#"]
You can replace the filter function above with:
languages = languages.filter(language => language !== needle) //ES6
We hope this blog post has helped you understand JavaScript arrays and how they can be used in code. If it’s not clear, don’t worry! The examples we provided should help to clarify the process of removing one or many items from an array for use in your own projects. Remember that a good way to practice using new coding skills is by working on other tutorials with similar concepts so you’ll get more comfortable before tackling bigger tasks like web design or app development. Have fun learning about javascript arrays and happy coding!