AnimatedSize is a Flutter animation widget that allows to animate its child’s size during a period.
AnimatedSize doesn’t work well on animation reversing. This might be a bug.
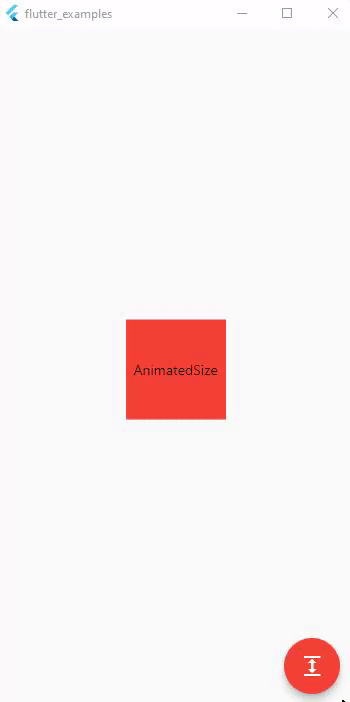
import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> with SingleTickerProviderStateMixin{ bool _expanded = false; @override Widget build(BuildContext context) { return Scaffold( body: Center( child: AnimatedSize( duration: Duration(seconds: 2), vsync: this, child: Container( width: _expanded ? double.infinity : 100, height: _expanded ? double.infinity : 100, color: Colors.red, child: Center(child: Text('AnimatedSize')), ), ), ), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { _expanded = !_expanded; }); }, backgroundColor: Colors.red, child: _expanded ? Icon(Icons.wrap_text_outlined) : Icon(Icons.expand), ), ); } @override void dispose() { _controller.dispose(); super.dispose(); } }