JavaScript has many built-in functions that can be used in your code to simplify repetitive tasks. Two of these are the slice() and substring() methods, which truncate a string so that it is a specific length or smaller. This article will show you how to use these functions to shorten strings in JavaScript and give you some examples on when to use it.
Table of Contents
slice()
The slice() method creates a deep copy of a portion of the array and returns it into a new array. The index for start is inclusive, but end is not. This method doesn’t modify the original array.
const wordLimit = 10; var phrase = "The JavaScript String is an object that contains a sequence of characters. Strings can be manipulated and used in many ways, but they are most often used with the indexOf method to find substrings within the string or at specific positions. The length property will return the number of characters in the string while methods like substring() and substr() provide more flexibility for finding and manipulating strings. More complex operations such as matching regular expressions against strings can also be performed using RegExp()."; var truncated = phrase.slice(0, wordLimit) + "..."; console.log(truncated); //output: "The JavaSc..."
substring()
The substring() method allows you to get the part of the string between two indices.
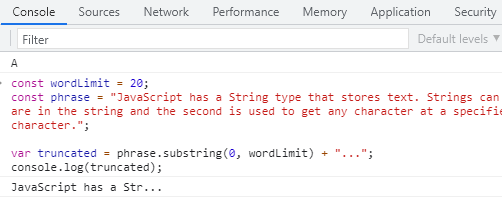
const wordLimit = 20; const phrase = "JavaScript has a String type that stores text. Strings can be created in many ways, but the most common are to use double quotation marks. All strings have two methods, length and charAt(). The first is used to get an integer of how many characters are in the string and the second is used to get any character at a specified index position. To access single characters from within a string you will need to use square brackets around your index number followed by curly braces for containing your desired character."; var truncated = phrase.substring(0, wordLimit) + "..."; console.log(truncated); //outout: "JavaScript has a Str..."