JavaScript is a programming language that is used to control the behavior of web pages. It can be used to make words capitalized or lowercase, and it can be used for many other things too. In this blog post, we will discuss how to capitalize a word in JavaScript. We will also go over some methods and examples.
There isn’t a built-in method to capitalize a word, so we have to use others and modify them to suit our required output. There are four methods we will use:
- toUpperCase() – This method capitalizes all the characters of a string and returns it in uppercase. To use this method, you need to call it on a string and store the result in a new variable.
- slice() – The slice()method extracts part of a string, returning it as another string without touching the original.
- charAt() – The String object’s charAt() method returns a new string consisting of the single UTF-16 code unit (a number between 0 and 65535) located at the specified offset into the string.
- toLowerCase() – The toLowerCase() method converts the string value passed into all lowercase letters.
- subsrtring() – The substring() method returns the part of the string between the start and end indexes, or the whole of it.
Here are some methods which can capitalize a word:
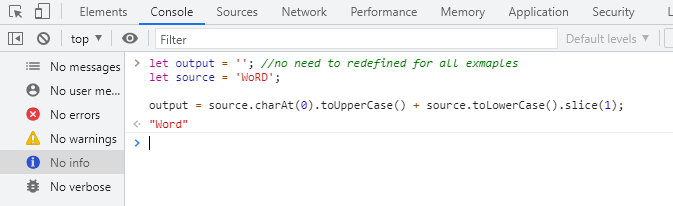
let output = ''; //no need to redefined for all exmaples let source = 'WoRD'; output = source.charAt(0).toUpperCase() + source.toLowerCase().slice(1); output = source[0].toUpperCase() + source.slice(1).toLowerCase(); output = source[0].toUpperCase() + source.substring(1).toLowerCase(); output = source.split('').map((character, index) => index ? character.toLowerCase() : character.toUpperCase()).join('');
let word = "javascript"; let capitalizedWord = word.toUpperCase(); console.log(capitalizedWord); // OUTPUT: JAVASCRIPT
let word = "javascript"; let capitalizedWord = word[0].toUpperCase() + word.slice(1); console.log(capitalizedWord); // OUTPUT: Javascript
You can also use regular expressions to capitalize the first letter of a string using a regular expression. To use this method, you need to create a regular expression pattern that matches the first letter of a string and call the replace()
method on it.
let word = "javascript"; let capitalizedWord = word.replace(/^\w/, function(match) { return match.toUpperCase(); }); console.log(capitalizedWord); // OUTPUT: Javascript