If you want to animate decoration property of a widget, you can use DecoratedBoxTransition. It is the animated version of DecoratedBox widget.
Besides using AnimatedContainer to animate a Container’s property, we can also use DecoratedBoxTransition.
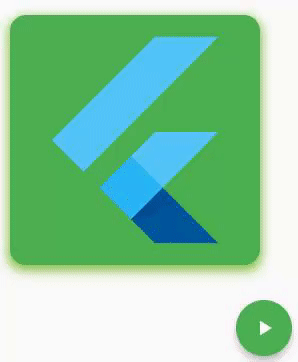
import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> with SingleTickerProviderStateMixin{ bool _play = false; final DecorationTween decorationTween = DecorationTween( begin: BoxDecoration( color: Colors.green, border: Border.all(style: BorderStyle.none), borderRadius: BorderRadius.circular(16.0), shape: BoxShape.rectangle, boxShadow: const <BoxShadow>[ BoxShadow( color: Colors.lightGreen, blurRadius: 8.0, spreadRadius: 2.0, offset: Offset(0, 4.0), ) ], ), end: BoxDecoration( color: Colors.blue, border: Border.all( style: BorderStyle.none, ), borderRadius: BorderRadius.zero, // No shadow. ), ); late final AnimationController _controller = AnimationController( vsync: this, duration: const Duration(seconds: 2), ); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Container( color: Colors.white, child: DecoratedBoxTransition( position: DecorationPosition.background, decoration: decorationTween.animate(_controller), child: Container( width: 250, height: 250, padding: const EdgeInsets.all(10), child: const FlutterLogo(), ), ), ), ), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { _play = !_play; }); if(_controller.status == AnimationStatus.completed){ _controller.reverse(); } else{ _controller.forward(); } }, backgroundColor: Colors.green, child: _play ? Icon(Icons.refresh) : Icon(Icons.play_arrow), ), ); } @override void dispose() { _controller.dispose(); super.dispose(); } }