AnimatedPositioned is an animated version of Positioned widget. It can transition the child’s position when there is a change in its child’s position values.
The widget requires to be a child of Stack widget to make it work.
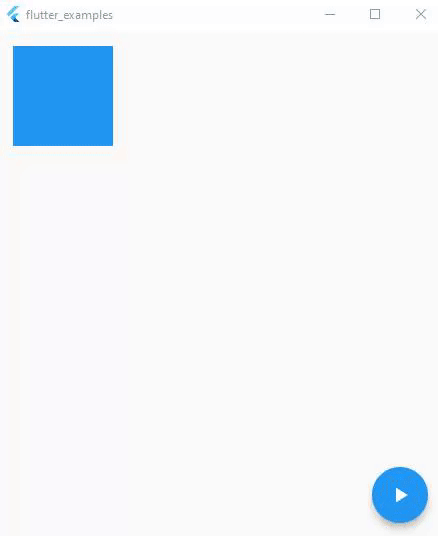
import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> { bool _play = false; @override Widget build(BuildContext context) { return Scaffold( body: Padding( padding: const EdgeInsets.all(16.0), child: Stack( children: [ AnimatedPositioned( top: 0, left: _play ? MediaQuery.of(context).size.width - 150 : 0, child: Container(width: 100, height: 100, color: Colors.red), duration: Duration(seconds: 1)), AnimatedPositioned( top: _play ? MediaQuery.of(context).size.width / 2 - 50 : 0, left: _play ? MediaQuery.of(context).size.width / 2 - 50 : 0, child: Container(width: 100, height: 100, color: Colors.green), duration: Duration(seconds: 1)), AnimatedPositioned( top: _play ? MediaQuery.of(context).size.height - 150 : 0, left: 0, child: Container(width: 100, height: 100, color: Colors.blue), duration: Duration(seconds: 1)), ], ), ), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { _play = !_play; }); }, child: Icon(Icons.play_arrow), ), ); } }