AnimatedCrossFade widget in Flutter can cross-faded between two given children and animates itself between their sizes. Basically, it provides fade transition effects between two widgets.
Table of Contents
AnimatedCrossFade
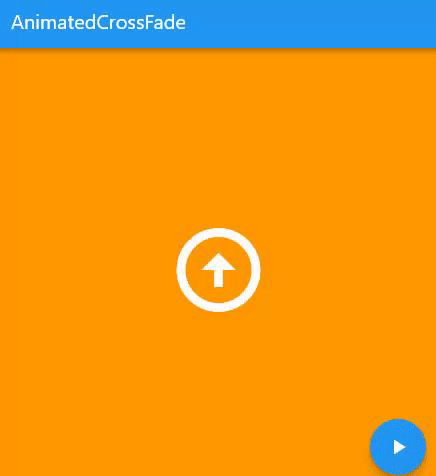
import 'dart:math'; import 'package:flutter/material.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { bool _isFirst = true; @override void initState() { super.initState(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('AnimatedCrossFade'), ), body: SafeArea( child: Container( color: Colors.orange, child: Center( child: AnimatedCrossFade( crossFadeState: _isFirst ? CrossFadeState.showFirst : CrossFadeState.showSecond, duration: const Duration(seconds: 1), firstCurve: Curves.easeInCubic, secondCurve: Curves.easeInCirc, firstChild: Icon(Icons.arrow_circle_up, size: 100, color: Colors.white), secondChild: Icon(Icons.arrow_circle_down, size: 100, color: Colors.white), )))), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { _isFirst = !_isFirst; }); }, child: Icon(Icons.play_arrow, color: Colors.white), ), ); } }
Important Properties:
Widget firstChild
: The child widget to be shown whencrossFadeState
isCrossFadeState.showFirst
.Widget secondChild
: The child widget to be shown whencrossFadeState
isCrossFadeState.showSecond
.CrossFadeState crossFadeState
: It accepts value between .CrossFadeState.showFirst and CrossFadeState.showSecond to determine which child to fade to.Duration duration
: Animation duration.Duration reverseDuration
: Reverse animation duration.
AnimatedCrossFade with layoutBuilder
layoutBuilder property is a builder for positioning the first child and the second child.
import 'dart:math'; import 'package:flutter/material.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { bool _isFirst = true; @override void initState() { super.initState(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('AnimatedCrossFade'), ), body: SafeArea( child: Container( color: Colors.orange, child: Center( child: AnimatedCrossFade( crossFadeState: _isFirst ? CrossFadeState.showFirst : CrossFadeState.showSecond, duration: const Duration(seconds: 1), firstCurve: Curves.easeInCubic, secondCurve: Curves.easeInCirc, firstChild: Icon(Icons.arrow_drop_up, size: 100, color: Colors.blue), secondChild: Icon(Icons.arrow_drop_down, size: 100, color: Colors.blue), layoutBuilder: (Widget topChild, Key topChildKey, Widget bottomChild, Key bottomChildKey) { return Stack( fit: StackFit.loose, children: <Widget>[ Positioned( key: bottomChildKey, left: 0.0, top: 0.0, right: 0.0, child: bottomChild, ), Positioned( key: topChildKey, child: topChild, ) ], ); }, )))), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { _isFirst = !_isFirst; }); }, child: Icon(Icons.play_arrow, color: Colors.white), ), ); } }