Splash and Introduction screens are kind of important in an app. While splashing screen helps users notify our the developer’s brand, introduction one helps user understand about the app and how to use some major features of the app.
Following are the best Flutter splash and introduction screen libraries:
Table of Contents
splashscreen
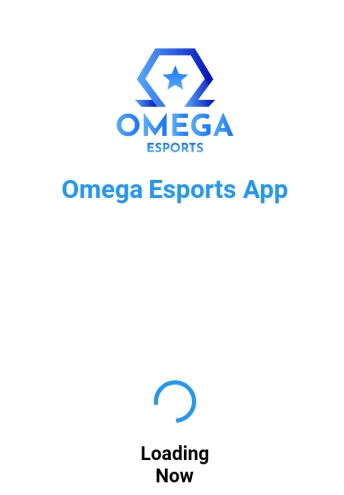
This package comes with a lot of customization, including appearing period, page to load after splash is completed, background color, image for app/company logo, and many more.
SplashScreen( seconds: 14, navigateAfterSeconds: HomePage(), title: Text('App title'), image: Image.asset('my_logo.png'), backgroundColor: Colors.red, styleTextUnderTheLoader: TextStyle(), photoSize: 300.0, loaderColor: Colors.red )
intro_views_flutter
intro_views_flutter can help developers create an app intro screens with some cool animations. It is designed to allow addition of pages and animation such as circular page reveal easily. Other minor features like skip button, animation control are also included to make it a good choice for creating introduction screens.
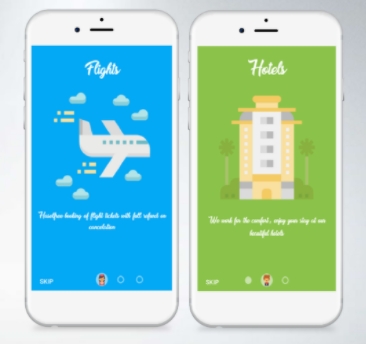
//define pages final pageOne = new PageViewModel( pageColor: Colors.blue, iconImageAssetPath: 'assets/airplane.png', iconColor: null, bubbleBackgroundColor: null, body: Text( 'Hello user', ), title: Text('Cabs'), mainImage: Image.asset( 'assets/airplane.png', height: 285.0, width: 285.0, alignment: Alignment.center, ), titleTextStyle: TextStyle(fontFamily: 'MyFont', color: Colors.white), bodyTextStyle: TextStyle(fontFamily: 'MyFont', color: Colors.white), ); final pageTwo = new PageViewModel( pageColor: Colors.blue, iconImageAssetPath: 'assets/building.png', iconColor: null, bubbleBackgroundColor: null, body: Text( 'The app can do it like this', ), title: Text('Cabs'), mainImage: Image.asset( 'assets/building.png', height: 285.0, width: 285.0, alignment: Alignment.center, ), titleTextStyle: TextStyle(fontFamily: 'MyFont', color: Colors.white), bodyTextStyle: TextStyle(fontFamily: 'MyFont', color: Colors.white), ); //add to slider final Widget introViews = new IntroViewsFlutter( [pageOne, pageTwo], onTapDoneButton: (){ }, showSkipButton: true, pageButtonTextStyles: new TextStyle( color: Colors.white, fontSize: 18.0, fontFamily: "Regular", ), );
intro_slider
intro_slider helps you make a cool intro for your app. Every property can be customized to make the slider suit your app.
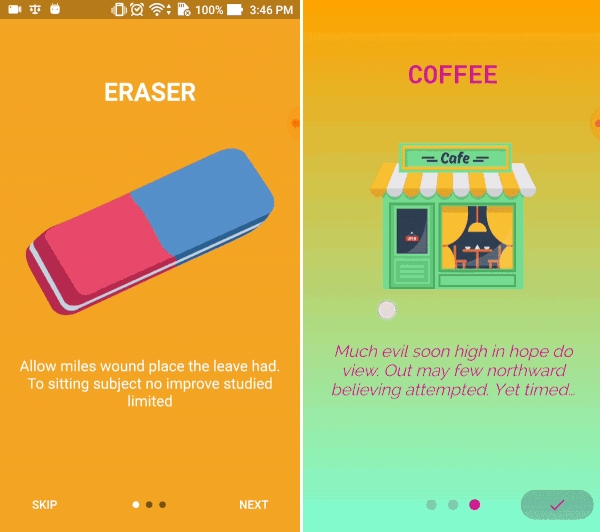
Define each slide
List<Slide> slides = new List(); slides.add( new Slide( title: "SCHOOL", styleTitle: TextStyle( color: Color(0xff3da4ab), fontSize: 30.0, fontWeight: FontWeight.bold, fontFamily: 'RobotoMono'), description: "Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Aenean commodo ligula eget dolor. Aenean massa.", styleDescription: TextStyle( color: Color(0xfffe9c8f), fontSize: 20.0, fontStyle: FontStyle.italic, fontFamily: 'Raleway'), pathImage: "images/photo_school.png", ), ); slides.add( new Slide( title: "MUSEUM", styleTitle: TextStyle( color: Color(0xff3da4ab), fontSize: 30.0, fontWeight: FontWeight.bold, fontFamily: 'RobotoMono'), description: "Ye indulgence unreserved connection alteration appearance", styleDescription: TextStyle( color: Color(0xfffe9c8f), fontSize: 20.0, fontStyle: FontStyle.italic, fontFamily: 'Raleway'), pathImage: "images/photo_museum.png", ), ); slides.add( new Slide( title: "COFFEE SHOP", styleTitle: TextStyle( color: Color(0xff3da4ab), fontSize: 30.0, fontWeight: FontWeight.bold, fontFamily: 'RobotoMono'), description: "Much evil soon high in hope do view. Out may few northward believing attempted. Yet timed being songs marry one defer men our. Although finished blessing do of", styleDescription: TextStyle( color: Color(0xfffe9c8f), fontSize: 20.0, fontStyle: FontStyle.italic, fontFamily: 'Raleway'), pathImage: "images/photo_coffee_shop.png", ), );
Add pages to slider
IntroSlider( // List slides slides: this.slides, // Skip button renderSkipBtn: this.renderSkipBtn(), colorSkipBtn: Color(0x33ffcc5c), highlightColorSkipBtn: Color(0xffffcc5c), // Next button renderNextBtn: this.renderNextBtn(), // Done button renderDoneBtn: this.renderDoneBtn(), onDonePress: this.onDonePress, colorDoneBtn: Color(0x33ffcc5c), highlightColorDoneBtn: Color(0xffffcc5c), // Dot indicator colorDot: Color(0xffffcc5c), sizeDot: 13.0, typeDotAnimation: dotSliderAnimation.SIZE_TRANSITION, // Tabs listCustomTabs: this.renderListCustomTabs(), backgroundColorAllSlides: Colors.white, refFuncGoToTab: (refFunc) { this.goToTab = refFunc; }, // Show or hide status bar shouldHideStatusBar: true, // On tab change completed onTabChangeCompleted: this.onTabChangeCompleted, )
nice_intro
Onboarding is a great way to introduce your app to newcomers, to help them quickly know what to do and where to carry out a task on your app.
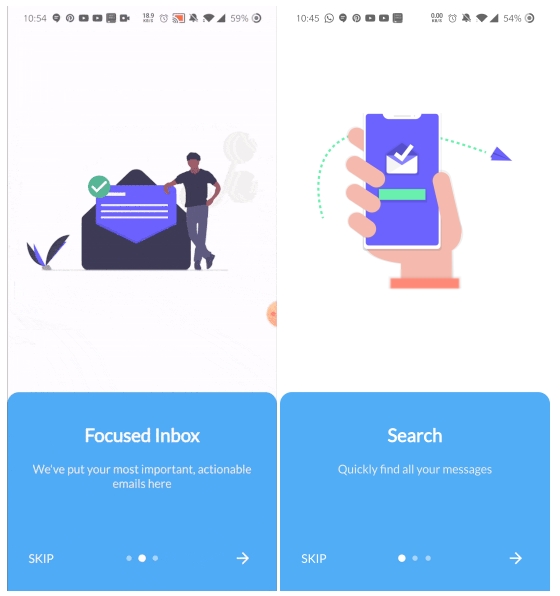
List<IntroScreen> pages = [ IntroScreen( title: 'Search', imageAsset: 'assets/img/1.png', description: 'Quickly find all your messages', headerBgColor: Colors.white, ), IntroScreen( title: 'Focused Inbox', headerBgColor: Colors.white, imageAsset: 'assets/img/2.png', description: "We've put your most important, actionable emails here", ), IntroScreen( title: 'Social', headerBgColor: Colors.white, imageAsset: 'assets/img/3.png', description: "Keep talking with your mates", ), ]; IntroScreens introScreens = IntroScreens( footerBgColor: TinyColor(Colors.blue).lighten().color, activeDotColor: Colors.white, footerRadius: 18.0, indicatorType: IndicatorType.CIRCLE, pages:pages )