Jiffy is a Dart date time package for parsing, manipulating, querying and formatting dates.
Install the package
jiffy: ^5.0.0
Table of Contents
Basic usage
//create Jiffy object from string or map Jiffy("2021-03-25"); // A calendar date part Jiffy("2021/03/25"); // A calendar date part separated by slash "/" Jiffy("20210325"); // Basic (short) full date Jiffy("2021-03-25 03:00:00.000"); // An hour, minute, second, and millisecond time part Jiffy("2021-03-25T03:00:00.000"); // ISO dart format Jiffy("2021-03-25T03:00:00.000Z"); // ISO dart format (UTC) Jiffy({ "hour": 19, "minutes": 20 }); Jiffy({ "year": 2019, "month": 10, "day": 19, "hour": 19 }); Jiffy([2021]); // January 1st Jiffy([2021, 03]); // March 1st Jiffy([2021, 09, 18]); // September 18th //get data Jiffy().milliseconds; Jiffy().seconds; Jiffy().minute; Jiffy().hour; Jiffy().date; Jiffy().daysInMonth; Jiffy().day; Jiffy().dayOfYear; Jiffy().week; Jiffy().month; Jiffy().quarter; Jiffy().year; //some methods Jiffy().add(years: 1); Jiffy().add(months: 3, duration: Duration(days: 3)); Jiffy().subtract(days: 3); Jiffy().startOf(Units.YEAR); Jiffy().endOf(Units.DAY); var jiffy1 = Jiffy("2008-10", "yyyy-MM"); var jiffy2 = Jiffy("2007-1", "yyyy-MM"); jiff1.diff(jiffy2, Units.YEAR); // 1 jiff1.diff(jiffy2, Units.YEAR, true); // 1.75 //show datetime Jiffy().format("MMMM do yyyy, h:mm:ss a");
Show current time every 1 second
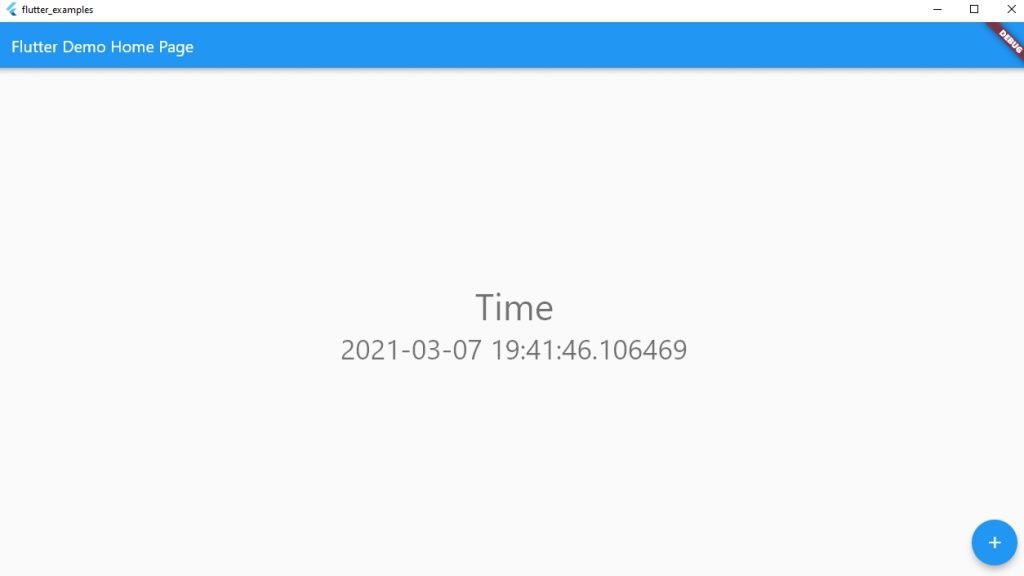
import 'dart:async'; import 'package:flutter/material.dart'; import 'package:jiffy/jiffy.dart'; class MyHomePage extends StatefulWidget { MyHomePage({Key key, this.title}) : super(key: key); final String title; @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { Timer timer; String time = ''; void _showTime() { setState(() { time = Jiffy().dateTime.toString(); }); } @override void initState() { super.initState(); _showTime(); timer = Timer.periodic(Duration(seconds: 1), (_) => _showTime()); } @override void dispose() { timer?.cancel(); super.dispose(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( 'Time', style: Theme.of(context).textTheme.headline3, ), Text( time, style: Theme.of(context).textTheme.headline4, ), ], ), ), floatingActionButton: FloatingActionButton( onPressed: _showTime, tooltip: 'Show', child: Icon(Icons.add), ), // This trailing comma makes auto-formatting nicer for build methods. ); } }