Odoo doesn’t support popup messages out of the box. There is this raise Warning(message)
which opens a popup to show errors. However, you SHOULD never use them to show messages to users.
In Odoo, everything runs inside a big transaction. When there is a raised exception, Odoo will carry out a rollback in the database for all committed transactions. So it is not recommended to use this approach to show informational or success messages.
Our correct approach is to use a wizard.
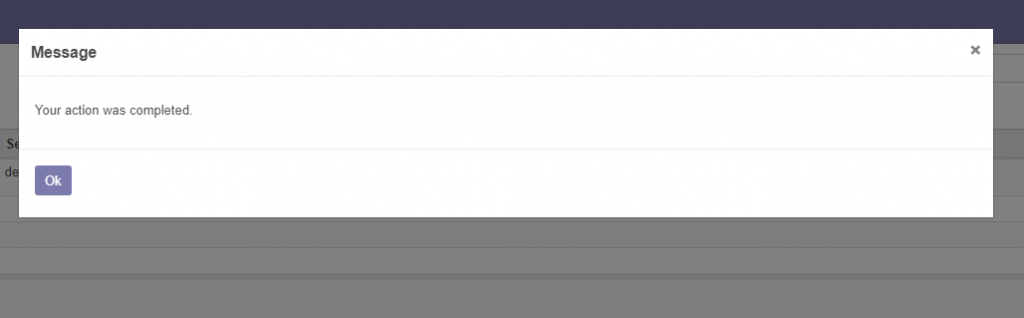
Wizard class
class MyModuleMessageWizard(models.TransientModel):
_name = 'mymodule.message.wizard'
_description = "Show Message"
message = fields.Text('Message', required=True)
@api.multi
def action_close(self):
return {'type': 'ir.actions.act_window_close'}
View
<!--Message Wizard -->
<record id="mymodule_message_wizard_form" model="ir.ui.view">
<field name="name">mymodule.message.wizard.form</field>
<field name="model">mymodule.message.wizard</field>
<field name="arch" type="xml">
<form>
<field name="message" readonly="True"/>
<footer>
<button name="action_close" string="Ok" type="object" default_focus="1" class="oe_highlight"/>
</footer>
</form>
</field>
</record>
Then call the wizard from any actions.
@api.multi
def mymodule_open_popup(self):
mymodule = self.get_server_info(self.name)
message_id = self.env['mymodule.message.wizard'].create({'message': 'Your action was completed.'})
return {
'name': 'Message',
'type': 'ir.actions.act_window',
'view_mode': 'form',
'res_model': 'mymodule.message.wizard',
'res_id': message_id.id,
'target': 'new'
}
There is no @api.multi in odoo15 version
It there alternative?
@api.multi is considcered as default behaviour since Odoo 13, so you don’t need the @api.multi decoration, just strating from the method.