The jQuery find method is one of the most powerful tools in any developer’s toolbox. It can be used to locate an element based on its ID, class name or tag name and has many other options for advanced searches. But how do you use it to find a parent element?
jQuery offers many methods to help developer spot an element’s parent or parents. In this post, we will differentiate these functions in order to use them better.
Table of Contents
closest() vs parents() vs parent() vs parentsUntil()
The ancestor can be any level like parent, grandparent, etc…
closest() | parent() | parents() | parentsUntil() |
---|---|---|---|
Returns the nearest parent element of the selector | Returns the direct parent element of the selector | Returns all ancestor elements of the selector | Returns all ancestors between the selector and stop |
Begins with the selector and traverses upward to find the frist parent | Begins with the selector and traverses upward to find the direct parent | Begins with the selector’s direct parent and traverses upward to find all parents which match the condition | Begins with the selector and traverses upward to find all parents until the stop condition |
Its returned object contains 0 or 1 element | Its returned object contains 0 or 1 element | Its returned object contains 0 or more than 1 element | Its returned object contains 0 or more than 1 element |
Examples
HTML tags
<div class="container"> <div class="header" style="padding: 16px;"> <nav style="padding: 16px;"> <ul> <li>Item #1</li> <li>Item #2</li> <li>Item #3</li> <li>Item #4</li> <li>Item #5</li> <li>Item #6</li> </ul> </nav> </div> </div>
closest()
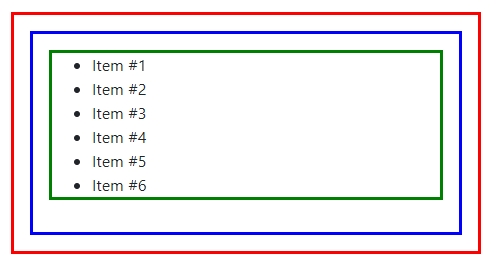
$(function(){ $("li").closest("ul").css({"border": "3px solid green"}); }); $(function(){ $("li").closest("nav").css({"border": "3px solid blue"}); }); $(function(){ $("li").closest("div").css({"border": "3px solid red"}); });
parent()
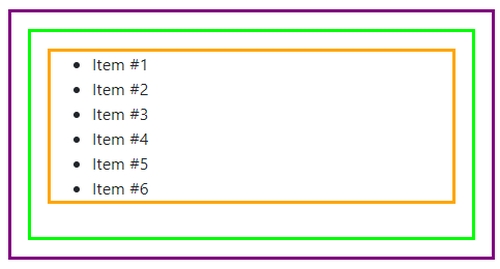
$(function(){ $("li").parent().css({"border": "3px solid orange"}); $("ul").parent().css({"border": "3px solid lime"}); $("nav").parent().css({"border": "3px solid purple"}); });
parents()
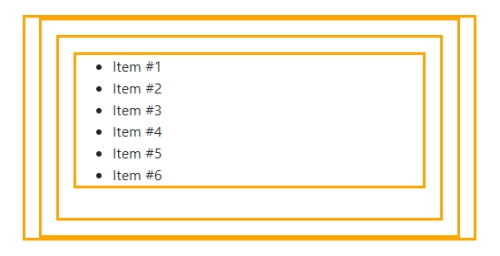
$(function(){ $("li").parents().css({"border": "3px solid orange"}); });
parentsUntil()
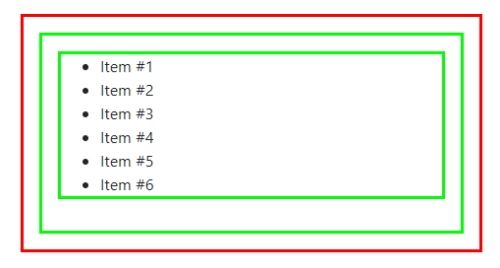
$(function(){ $('.header').css({"border": "3px solid red"}); $("li").parentsUntil('.header').css({"border": "3px solid lime"}); });