In most UI/UX you will see image getting displayed in a circular shape. In this post, we are going to create a circle image in a Flutter app.
Below is how to create a circle image downloading from an URL. You can change it to other image sources such as Image.asset
or Image.file
.
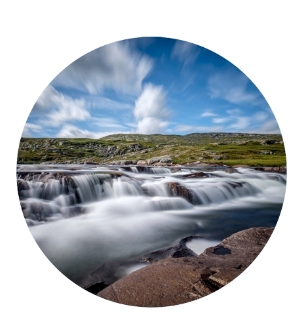
class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: MainAppBar(title: 'Home'), backgroundColor: Colors.white, body: SafeArea( child: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ Row( mainAxisAlignment: MainAxisAlignment.center, children: [ Container( decoration: BoxDecoration(color: Colors.blue, shape: BoxShape.circle), height: 300, width: 300, child: ClipOval( child: Image.network( "https://cdn.pixabay.com/photo/2018/10/04/11/31/river-3723439_960_720.jpg", fit: BoxFit.fitHeight,), ), ), ], ) ], ), ), ); } }