It is important to format numbers with leading zeros in PHP. This will ensure that the number is formatted correctly. Numbers with leading zeros are much easier to read and understand than numbers without them.
The formatting of a number can make or break a project when it comes to presenting data professionally. Luckily there are many different methods and formats available! In this article we’ll cover some basics about how you can easily format your numbers with leading zeros.
There are 3 ways to add leading zeroes to an integer in PHP – str_pad
, printf/sprintf
, and substr
.
Table of Contents
str_pad()
str_pad() function pads a string to a certain length with another string. The function will pad a string to the left or right with another specified character. It is important that you use all four arguments for this one if you want to pad left side.
echo str_pad('15', 3, "0", STR_PAD_LEFT);
//output: 015
printf()/sprintf()
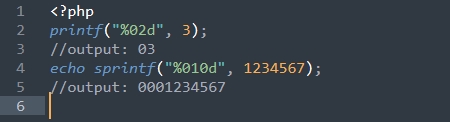
You can also use the these string formatting functions to format numbers, “rounding functions,” and currency conversion.
A number can be formatted in PHP with leading zeros by using the printf() function. This will provide a more professional appearance to your numbers. The syntax is as follows: printf("%02d", $number);
.
sprintf() is a PHP method which will automatically convert a number input to a string and pad it with zeros accordingly.
printf("%02d", 3);
//output: 03
echo sprintf("%010d", 1234567);
//output: 0001234567
substr()
substr() in PHP can be used to extract a substring out of a given string. It can be accessed using the following syntax: substr($string, start_position, end_positon)
.
The start position is specified as an integer with respect to $string and denotes where in the string we want to start extracting from. The end position specifies how many characters or how many characters followed by the end of string.
To add leading zeroes to a number, we combine str_repeat and substr to repeat number of 0 at the beginning of the given number.
$number = 100; $length = 5; $result = substr(str_repeat(0, $length).$number, - $length); //output: 00100
str_repeat() returns string repeated a number of times.
In this article we’ve covered some of the basics about how to easily format your numbers with leading zeros. Whether you’re printing out a report, making a spreadsheet or writing an email – these formatting tips will help make sure that what you present is professional and easy for others to read!