In JavaScript the getElementsByClassName()
the method returns an HTMLCollection object which is a collection of all elements in the document with the class name parameter in the method.
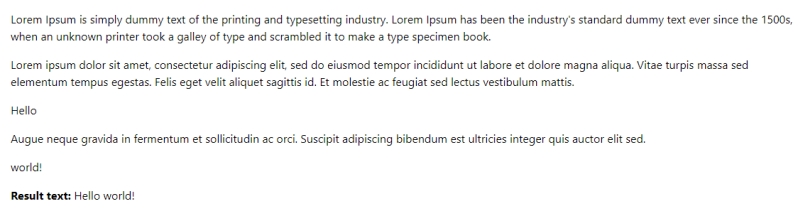
The HTMLCollection object is a collection of nodes, which can be accessed by index numbers. As a result, you can use for loop to Iterate through the getElementsByClassName() result.
HTML
<p>Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book.</p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Vitae turpis massa sed elementum tempus egestas. Felis eget velit aliquet sagittis id. Et molestie ac feugiat sed lectus vestibulum mattis.</p> <p class="text">Hello</p> <p>Augue neque gravida in fermentum et sollicitudin ac orci. Suscipit adipiscing bibendum est ultricies integer quis auctor elit sed.</p> <p class="text">world!</p> <p><b>Result text:</b> <span class="result"></span></p>
JavaScript: use for
loop
var texts = document.getElementsByClassName("text"); for (var i = 0; i < texts.length; i++) { var result = document.getElementsByClassName("result")[0]; result.innerHTML += texts[i].innerText + ' '; }
JavaScript: use querySelectorAll
loop
document.querySelectorAll('.text').forEach(function(text) { var result = document.getElementsByClassName("result")[0]; result.innerHTML += text.innerText + ' '; });