FadeTransition is a neat Flutter widget that animates the opacity of its child. This widget can be set with a fading animation to reveal or hide a widget.
To change the animation for a child widget, you must pass in an Animation<double> object to define what needs to be applied.
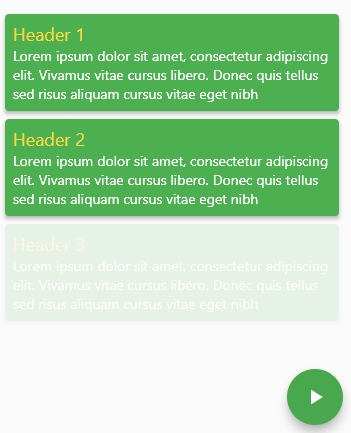
import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> with SingleTickerProviderStateMixin{ late final AnimationController _controller = AnimationController( vsync: this, duration: const Duration(seconds: 2), ); late final Animation<double> _animation1 = CurvedAnimation( parent: _controller, curve: Interval( 0.0, 0.4, curve: Curves.easeInCirc, ), ); late final Animation<double> _animation2 = CurvedAnimation( parent: _controller, curve: Interval( 0.4, 0.8, curve: Curves.easeInCirc, ), ); late final Animation<double> _animation3 = CurvedAnimation( parent: _controller, curve: Interval( 0.8, 1, curve: Curves.easeInCirc, ), ); FadeTransition _card(int index, Animation<double> animation){ return FadeTransition( opacity: animation, child: Card( elevation: 3, color: Colors.green, child: Container( padding: EdgeInsets.all(8.0), width: double.infinity, child: Column( mainAxisAlignment: MainAxisAlignment.start, crossAxisAlignment: CrossAxisAlignment.start, children: [ Text('Header $index', style: TextStyle(fontSize: 18.0, color: Colors.amberAccent)), Text('Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vivamus vitae cursus libero. Donec quis tellus sed risus aliquam cursus vitae eget nibh', style: TextStyle(color: Colors.white)), ], ), ), ), ); } @override Widget build(BuildContext context) { return Scaffold( body: Padding( padding: const EdgeInsets.all(16.0), child: Column( mainAxisAlignment: MainAxisAlignment.start, crossAxisAlignment: CrossAxisAlignment.start, children: [ _card(1, _animation1), _card(2, _animation2), _card(3, _animation3), ], ), ), floatingActionButton: FloatingActionButton( onPressed: () { if(_controller.status == AnimationStatus.completed){ _controller.reverse(); } else{ _controller.forward(); } }, backgroundColor: Colors.green, child: Icon(Icons.play_arrow), ), ); } @override void dispose() { _controller.dispose(); super.dispose(); } }