HTML select field is a common way to get user input. However, when you need the field to be read-only, it can become difficult because users can still change the value of a selection using the select field’s arrow despite the value is not changed. This blog post will show you how to stop this from happening without having any negative consequences on your user experience.
Select fields in HTML are typically used for a user to pick one or more options. These can be inputted via the keyboard, mouse, or touchscreen. But what if you want to prevent the option from being changed? There are many ways to block people from changing things in your HTML select field. You can use JavaScript, jQuery, or CSS.
Let’s use different approaches to handle the HTML form below:
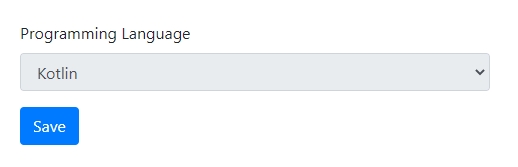
<form> <div class="form-group"> <label>Programming Language</label> <select class="form-control" readonly> <option value="kotlin">Kotlin</option> <option value="java">Java</option> <option value="flutter">Flutter</option> <option value="reactnative">React Native</option> </select> </div> <div class="form-group"> <input type="submit" name="submit" class="btn btn-primary" value="Save" /> </div> </form>
Table of Contents
Using CSS
Users can still select a read-only select because of the arrow on the right side of the field. We can just use CSS to disable the click events of the arrow.
select[readonly] { pointer-events: none; }
When the user presses Tab to switch fields in a form, they can still trigger the drop-down event. That’s why in the HTML code of the select field, we need to set its tabindex to -1.
<select class="form-control" readonly tabindex="-1"> ...
Using JavaScript
This approach sets the select field’s state in 2 steps.
- When the field is first loaded, set its state to
disabled
to prevent user interaction. - When the form is submitted, remove the
disabled
state to allow its value to be passed.
<select class="form-control" disabled="disabled"> ...
document.querySelector("form").addEventListener("submit", function(e){ document.getElementsByTagName("select")[0].removeAttribute("disabled"); });
Using jQuery
It is the same as the JavaScript method but switches the language to jQuery for those who love to use this framework.
$(function(){ $('form').on('submit', function(){ $('select').removeAttr('disabled'); }); });