Bluetooth technology has radically changed how we connect devices together, and mobile app development is no exception. In many cases, developers need to work with Bluetooth communication such as connecting to a printer or a headphone. Following are the best Flutter Bluetooth packages.
To use Bluetooth in either Android or iOS, you need to add permissions to AndroidManifest.xml
or Info.plist
.
//Android <uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/> //iOS <dict> <key>NSBluetoothAlwaysUsageDescription</key> <string>Need BLE permission</string> <key>NSBluetoothPeripheralUsageDescription</key> <string>Need BLE permission</string> <key>NSLocationAlwaysAndWhenInUseUsageDescription</key> <string>Need Location permission</string> <key>NSLocationAlwaysUsageDescription</key> <string>Need Location permission</string> <key>NSLocationWhenInUseUsageDescription</key> <string>Need Location permission</string>
Table of Contents
flutter_blue
FlutterBlue helps connect and communicate with Bluetooth Low Energy devices. It supports Android and iOS. This package is under active development, so there might be break changes in an upcoming version.
bluetooth bluetooth = bluetooth.instance; //scan and find Bluetooth devices bluetooth.startScan(timeout: Duration(seconds: 6)); var subscription = bluetooth.scanResults.listen((results) { // do something with scan results for (ScanResult r in results) { print('${r.device.name} found! rssi: ${r.rssi}'); } }); bluetooth.stopScan();
flutter_ble_lib
flutter_ble_lib is a Flutter library that works with Bluetooth Low Energy. It supports BLEmulator, a BLE simulator, making it easier to develop Bluetooth apps.
BleManager bleManager = BleManager(); await bleManager.createClient(); BluetoothState currentState = await bleManager.bluetoothState(); bleManager.observeBluetoothState().listen((btState) { print(btState); }); bleManager.destroyClient();
blue_thermal_printer
This Flutter plugin allows users to connect to a thermal printer through Bluetooth on Android devices. It is currently in the development stage, so it is not yet available for use. This plugin will enable users to send text, images, and other data to the printer, allowing them to easily print documents, tickets, receipts, and more.
BlueThermalPrinter bluetooth = BlueThermalPrinter.instance; List<BluetoothDevice> devices = []; try { devices = await bluetooth.getBondedDevices(); } on PlatformException { print('Error'); } bluetooth.onStateChanged().listen((state) { switch (state) { case BlueThermalPrinter.CONNECTED: break; case BlueThermalPrinter.DISCONNECTED: break; default: print(state); break; } });
bluetooth_print
This plugin also allows a device to connect to a thermal printer. Furthermore, it supports both Android and iOS.
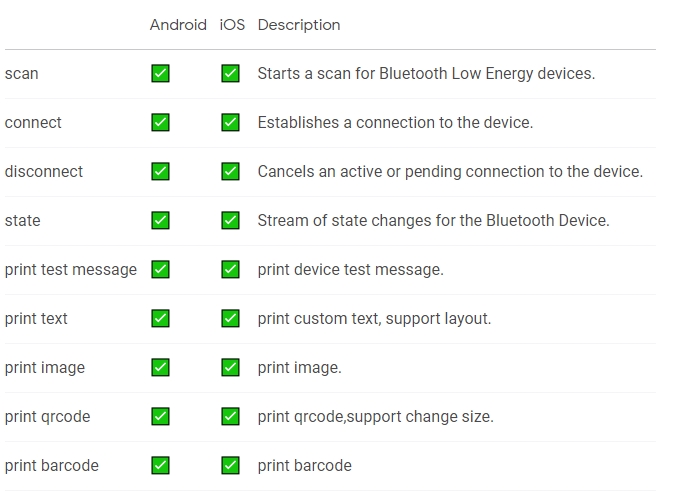
bluetooth bluetooth = bluetooth.instance; // scan and get devices bluetooth.startScan(timeout: Duration(seconds: 4)); // get devices StreamBuilder<List<BluetoothDevice>>( stream: bluetooth.scanResults, initialData: [], builder: (c, snapshot) => Column( children: snapshot.data.map((d) => ListTile( title: Text(d.name??''), subtitle: Text(d.address), onTap: () async { setState(() { _device = d; }); }, trailing: _device!=null && _device.address == d.address?Icon( Icons.check, color: Colors.green, ):null, )).toList(), ), ), //connect and disconnect a device await bluetooth.connect(_device); await bluetooth.disconnect(); //print Map<String, dynamic> config = Map(); config['width'] = 50; config['height'] = 60; config['gap'] = 3; List<LineText> data = List(); list.add(LineText(type: LineText.TYPE_TEXT, content: 'A Title', weight: 1, align: LineText.ALIGN_CENTER,linefeed: 1)); list.add(LineText(type: LineText.TYPE_TEXT, content: 'this is conent left', weight: 0, align: LineText.ALIGN_LEFT,linefeed: 1)); list.add(LineText(type: LineText.TYPE_TEXT, content: 'this is conent right', align: LineText.ALIGN_RIGHT,linefeed: 1)); list.add(LineText(linefeed: 1)); list.add(LineText(type: LineText.TYPE_BARCODE, content: 'A12312112', size:10, align: LineText.ALIGN_CENTER, linefeed: 1)); list.add(LineText(linefeed: 1)); list.add(LineText(type: LineText.TYPE_QRCODE, content: 'qrcode i', size:10, align: LineText.ALIGN_CENTER, linefeed: 1)); list.add(LineText(linefeed: 1)); await bluetooth.printReceipt(config, data);
quick_blue
This cross-platform BluetoothLE plugin for Flutter supports Android, iOS, macOS, and Windows. This is the most versatile Bluetooth plugin yet.
QuickBlue.setConnectionHandler(_handleConnectionChange); void _handleConnectionChange(String deviceId, BlueConnectionState state) { print('_handleConnectionChange $deviceId, $state'); } QuickBlue.connect(deviceId); QuickBlue.disconnect(deviceId);