Below are some libraries which can help display and cache image in Android. Each one has its own pros and cons so pick one carefully based on how complicated your project is.
Table of Contents
Picasso
Picasso is a simple yet powerful image downloading and caching library. I like using Picasso in all of my projects. The reason behind it is because this image library is the only one I knew when I first started learning Android development. I like how it allows for simple image loading in my app with only one line of code in most cases.
Picasso.with(context).load("https://www.tldevtech.com/wp-content/uploads/tdt/2016/05/logo.png").into(imageView1);
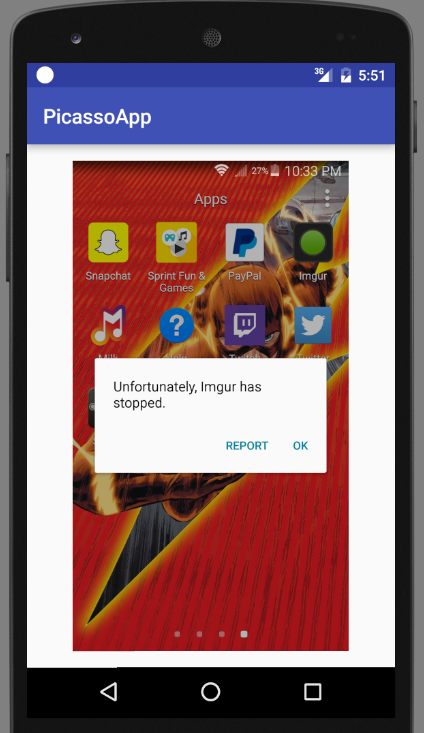
Universal Image Loader
Universal Image Loader’s purpose is to provide a powerful, flexible and highly customizable tool for image loading, displaying and caching. Universal Image Loader provides a lot of customizable options and good control over the image loading and caching process. Almost everything can be customized. This library caches image in memory and/or in internal storage/sdcard.
Below is a quick look at what options you can customize in Universal Image Loader.
DisplayImageOptions options = new DisplayImageOptions.Builder()
.showImageOnLoading(R.drawable.image_loading)
.showImageForEmptyUri(R.drawable.image_placeholder)
.showImageOnFail(R.drawable.image_error)
.resetViewBeforeLoading(false)
.delayBeforeLoading(1000)
.cacheInMemory(true)
.cacheOnDisk(true)
.preProcessor(...)
.postProcessor(...)
.extraForDownloader(...)
.considerExifParams(false)
.imageScaleType(ImageScaleType.IN_SAMPLE_POWER_OF_2)
.bitmapConfig(Bitmap.Config.ARGB_8888)
.decodingOptions(...)
.displayer(new SimpleBitmapDisplayer())
.handler(new Handler())
.build();
Glide
Glide is an image library that focuses on smooth scrolling. Glide can fetch, decode, and display images and animated GIFs fast and smooth. Glide is effective for almost any cases where you need to work with images downloaded from internet. Glide is similar to Picasso when it comes to simple code.
ImageView imageView = (ImageView) findViewById(R.id.imageView);
Glide.with(this).load("https://www.tldevtech.com/wp-content/uploads/tdt/2016/05/logo.png").into(imageView);
Fresco
Fresco is a powerful library to display images in Android app. Fresco can load images from an URL, local storage, or local resources. The library has 3 levels of cache that can reduce workload of CPU and data. Two levels are put in memory and the other is out in internal storage. That’s why apps using Fresco can run even on low-end devices without having to constantly keep image memory in check. Fresco uses a placeholder for an image until it is loaded and then the placeholder automatically shows the image.
Fresco also supports progressive JPEGs’ treaming, animated GIFs and WebPs, extensive customization of image loading and display and much more. It can also be used as a view.
<com.facebook.drawee.view.SimpleDraweeView
android:id="@+id/simpleDraweeView"
android:layout_width="50dp"
android:layout_height="50dp"
fresco:fadeDuration="500"
fresco:actualImageScaleType="focusCrop"
fresco:placeholderImage="@color/wait_color"
fresco:placeholderImageScaleType="fitCenter"
fresco:failureImage="@drawable/error"
fresco:failureImageScaleType="centerInside"
fresco:retryImage="@drawable/retrying"
fresco:retryImageScaleType="centerCrop"
fresco:progressBarImage="@drawable/progress_bar"
fresco:progressBarImageScaleType="centerInside"
fresco:progressBarAutoRotateInterval="1500"
fresco:backgroundImage="@color/blue"
fresco:overlayImage="@drawable/watermark"
fresco:pressedStateOverlayImage="@color/red"
fresco:roundAsCircle="false"
fresco:roundedCornerRadius="1dp"
fresco:roundTopLeft="true"
fresco:roundTopRight="false"
fresco:roundBottomLeft="false"
fresco:roundBottomRight="true"
fresco:roundWithOverlayColor="@color/corner_color"
fresco:roundingBorderWidth="2dp"
fresco:roundingBorderColor="@color/border_color"
/>
ion
Ion is an Android asynchronous networking and image loading library. It supports both memory and disk caching. Bitmaps are held via weak references so memory is managed very efficiently. Ion uses DeepZoom to allow images to be zoomed really large.
Ion.with(context)
.load("https://www.tldevtech.com/wp-content/uploads/tdt/2016/05/logo.png")
.withBitmap()
.placeholder(R.drawable.image_placeholder)
.error(R.drawable.image_error)
.animateLoad(spinAnimation)
.animateIn(fadeInAnimation)
.intoImageView(imageView);
Ion can read JSON data and download image from those data.
Ion.with(context)
.load("http://example.com/thing.json")
.asJsonObject()
.setCallback(new FutureCallback<JsonObject>() {
@Override
public void onCompleted(Exception e, JsonObject result) {
// do stuff with the result or error
}
});
Good aricle