Flutter is a UI toolkit, developed by Google, and you can use it to create a beautiful native app for desktop, mobile, and web. For faster development and native performance, you can choose Flutter for building an app. Hot Reload and customizable widgets are some notable features of Flutter. In this post, you will find the bets resources to learn Flutter, be it online courses, blogs, or detailed tutorials.
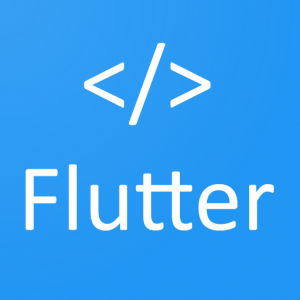
This Android app is a collection of widgets and animations with source code, all built with Flutter. You can learn the basics of Flutter widgets and find source codes for many Flutter design patterns.
Table of Contents
Dart Cheat Sheet
Understand Dart language before learning Flutter!
Declare variables
String website = 'TLTemplates'; final items = ['Item A', 'Item B', 'Item C']; const size = [200, 300]; //dynamic type can have value of any type var username; username = 'tester'; username = 10000;
Working with Null
//If b is not null, set a to b. Otherwise, set a to 0. a = b ?? 0; //if a is null, set 'default value' to a. a ??= 'default value'; //if a is not null, access property b of object a. a?.b //chain with property null check a?.b?.aMethod()
String interpolation
'${10 - 3}' //7 //The number's floor value is 100 double width = 100.2545; print('The number\'s floor value is ${width.floor()}'); //equivalent: someObject.toString() '$someObject' //escape quote with slash String message = 'John\'s car was fixed in 2019.'; //preserving format with ''' ''' This is a long message The 2nd line lies here ''' //raw string with r var str = r"This string won't decode \n or \t";
Control Flow
//if var a = 10; if(a > 0){ print('positive'); } else if(a == 0) { print('zero'); } else { print('negative'); } //when enum Type { normal, boost, enhance, reduce } final usedType = Type.normal; switch (usedType) { case Type.normal: break; case Type.boost: break; default: break; } //for loop for(var j = 0; j < 100; j++){ } //while, do-while loop var i = 0; while(i < 10){ i++; } int a = 10; do { a++; } //for each for(var type in Type){ print('$type'); }
Extension methods
extension <extension name> on <type> { (<member definition>)* } print(32.toAgeString()); extension AgeHandler on int { String toAgeString() { String text = this > 1 ? 'years old' : 'year old'; return '$this year(s) old'; } }
Collections
//create list of 100 integer from 0 to 99 List<int> items = List.generate(100, (index) => index); //loop for (var i in items) { print(i); } for(var i = 0; i < items.length; i++){ print(i); } //add item to list using if var cars = [ 'Huyndai', 'Madza', if (isJapan) 'Toyota' ]; //set var intSet = <int>{}; inSet.addAll([1, 2, 3, 4]); inSet.remove(2); inSet.add(5); //[1, 3, 4, 5] intSet.map((number) => number*2).toList(); //[2, 6, 8, 10] intSet.where((number) => number < 8); //[2, 6] var halogens = {'fluorine', 'chlorine', 'bromine', 'iodine', 'astatine'}; var intersection = setOne.intersection(secTwo); var union = setOne.union(secTwo); //map var strMap = Map<String, int>(); var gifts = { 'first': 'partridge', 'second': 'turtledoves', 'fifth': 'golden rings' }; gifts.keys.forEach(print); //'first', 'second', 'fifth' gifts.values.forEach(print); //'partridge', 'turtledoves', 'golden rings' gifts.forEach((key, value) => print('$key: $value'));
Class
//constructors Point(this.x, this.y); //normal constructor factory Point(int x, int y) => ...; //factory constructor Point.fromJson(Map json) { x = json['x']; y = json['y']; } //named constructor //getters and setters class MyClass { String job; String name; MyClass(String job, String name); String get display => '$name - $job'; set title(String value) { if (value.isNotEmpty) { job = value; } } } //inheritance class SubClass extends MyClass{ bool activated; SubClass(String job, String name) : super(job, name); } //chain methods //equivalent: user.age = 10;user.name = 'Tester' user..age = 10..name = 'Tester';
Flutter Cheat Sheet
Container
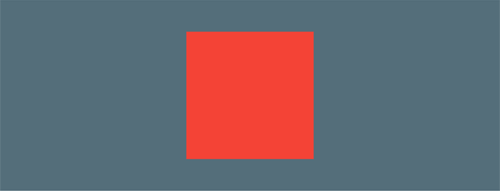
Container
Container( width: 100, height: 100, color: Colors.red, )
SizedBox
SizedBox( width: 100, height: 100, child: Container( color: Colors.red, ), )
ConstrainedBox
ConstrainedBox( constraints: BoxConstraints.expand(), child: Container( color: Colors.red, ))
Horizontal Layout
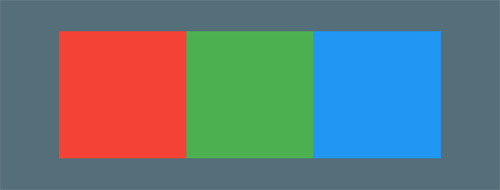
Row( mainAxisAlignment: MainAxisAlignment.center, children: [ Container( width: 100, height: 100, color: Colors.red, ), Container( width: 100, height: 100, color: Colors.green, ), Container( width: 100, height: 100, color: Colors.blue, ) ], )
Vertical Layout
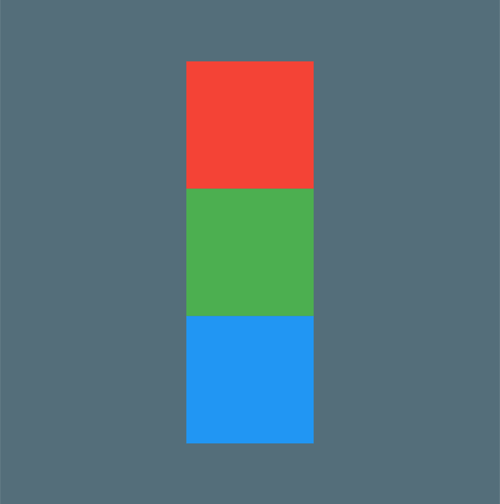
Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ Container( width: 100, height: 100, color: Colors.red, ), Container( width: 100, height: 100, color: Colors.green, ), Container( width: 100, height: 100, color: Colors.blue, ) ], )
Relative / Absolute Layout
Stack
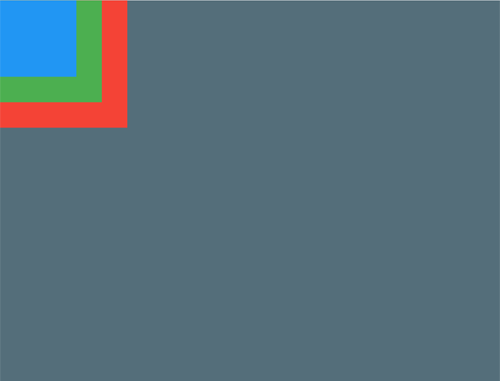
Stack( children: [ Container( width: 100, height: 100, color: Colors.red, ), Container( width: 80, height: 80, color: Colors.green, ), Container( width: 60, height: 60, color: Colors.blue, ) ], )
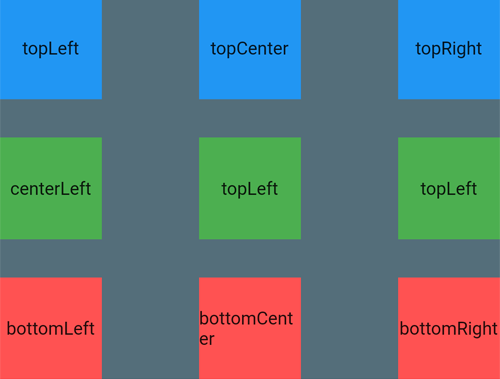
Align( alignment: Alignment.topLeft, child: Container( width: 80, height: 80, color: Colors.blue, child: Center(child: Text('topLeft')), ), )
Positioned
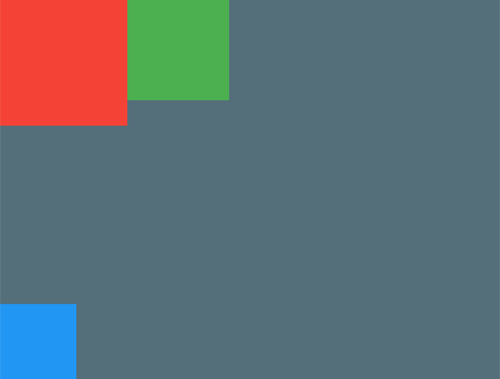
Stack( children: [ Positioned( child: Container( width: 100, height: 100, color: Colors.red, ), ), Positioned( left: 100, child: Container( width: 80, height: 80, color: Colors.green, ), ), Positioned( bottom: 0, child: Container( width: 60, height: 60, color: Colors.blue, ), ) ], )
Navigation
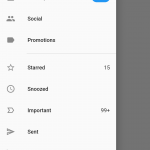
Scaffold( appBar: AppBar(title: Text('Drawer')), drawer: Drawer(), )

Scaffold( appBar: AppBar(title: Text('Bottom Navigation')), backgroundColor: Colors.blueGrey, bottomNavigationBar: BottomNavigationBar( items: [ BottomNavigationBarItem(icon: Icon(Icons.home), label: 'Home'), BottomNavigationBarItem(icon: Icon(Icons.search), label: 'Search'), BottomNavigationBarItem(icon: Icon(Icons.settings), label: 'Settings'), ], ), )
Item List
ListView
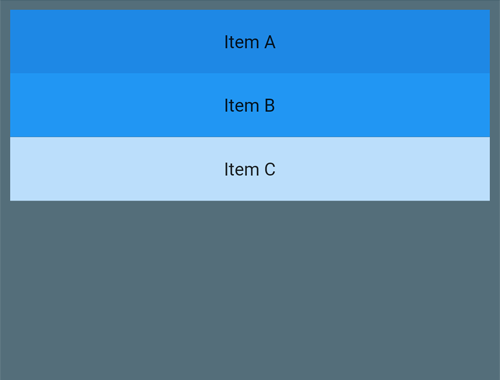
ListView( padding: const EdgeInsets.all(8), children: <Widget>[ Container( height: 50, color: Colors.blue[600], child: const Center(child: Text('Item A')), ), Container( height: 50, color: Colors.blue[500], child: const Center(child: Text('Item B')), ), Container( height: 50, color: Colors.blue[100], child: const Center(child: Text('Item C')), ), ], )
GridView
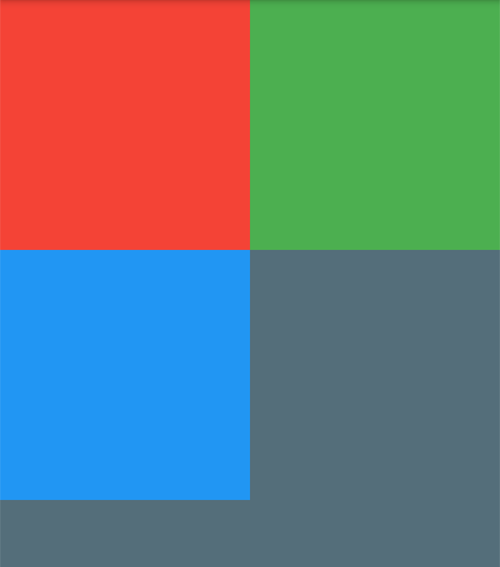
GridView.count( crossAxisCount: 2, children: <Widget>[ Container( color: Colors.red, ), Container( color: Colors.green, ), Container( color: Colors.blue, ), ], )
SliverList
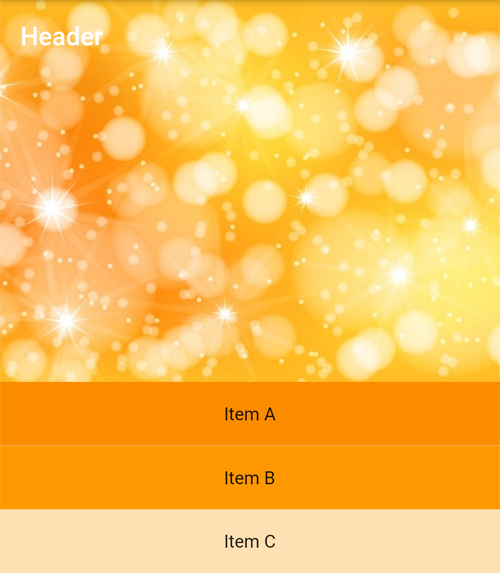
CustomScrollView( slivers: [ SliverAppBar( title: Text('Header'), backgroundColor: Colors.blue, expandedHeight: 300.0, flexibleSpace: FlexibleSpaceBar( background: Image.network('https://cdn.pixabay.com/photo/2017/12/13/16/40/background-3017167_960_720.jpg', fit: BoxFit.cover), ), ), SliverList( delegate: SliverChildListDelegate( [ Container( height: 50, color: Colors.orange[600], child: const Center(child: Text('Item A')), ), Container( height: 50, color: Colors.orange[500], child: const Center(child: Text('Item B')), ), Container( height: 50, color: Colors.orange[100], child: const Center(child: Text('Item C')), ), ], ), ) ] )
Learn Flutter with Tutorials and Courses
Online Courses and Basic Tutorials
- Flutter – Beginners Course – It is a comprehensive course for beginners with a guide on creating Flutter-based mobile apps. However, you have to understand the Dart language to join this course. The paid course at Udemy will enable you to develop apps with the Flutter framework. This is the best course to learn Flutter for beginners.
- Dart and Flutter: The Complete Developer’s Guide – Before digging into Flutter, one needs to know about Dart. This course covers Dart language and its primary features first, then teaches about Flutter’s screens, complex animations, network requests, react programming, etc…
- Flutter Development Bootcamp Using Dart – You can pay for this premium course, presenting you with a perfect curriculum on Flutter app development. You will know the technique of using Dart for code development. The tutorial guides you on creating the native-quality app. Thus, choose this online course to learn the Flutter setup and Flutter app development process.
- Flutter Succinctly – You can download this free e-book on your mobile and learn the details of Flutter app development. The e-book presents you with 129 pages with information on app fundamentals and app UI. You will learn the way of using customizable widgets to build the app.
- Flutter in Action – We have chosen it as the most reliable paid tutorial on Flutter. The book guides you on building professional mobile apps with the Dart programming language and Flutter SDK. You can find several annotations and diagrams that make your learning process easier. The tutorial also includes routing and other complicated topics.
- Flutter Tutorials – The free tutorial guides you on the way of using the Flutter framework to develop different apps. It also includes a layout mechanism, widgets (horizontal and vertical), and animation package of Flutter. You will learn tricks of internationalizing a Flutter application.
- Build A Chat Application With Firebase, Flutter and Provider – This Udemy course teaches you how to make a complete mobile messaging application like WhatsApp or Telegram using Flutter, Firebase and Provider Framework.
- Official Flutter Youtube Channel – This channel is a goto place to learn things about Flutter, including usage of different widgets, and Google IO videos content.
- Marcus Ng – He is a mobile developer who has published a lot of tutorials about how to create a design in Flutter.
- Login with flutter_bloc – Implement a login flow with username and pass using flutter_block.
- Tip Calculator – In this tutorial you will learn how to make a Tip Calculator. It is best to learn about form input and calculation.
- Todo app with flutter_bloc – Create your todo app, including create todo, todo list with checkbox.
- A complete guide to Flutter – This repo aims to guide new Flutter user through a huge list of working application using Flutter Web.
- Navigation done right – In this article, the developer briefly describes navigation principles used in one of his popular apps and how to implement it using just the framework-provided Navigator.
- WordPress Woocommerce app – If you want to learn about how to get data from WordPress’ Woocommerce shop and implement it as an app, you can check videos in this playlist.
- Suragch – A variety of articles about Flutter and Dart development with an emphasis in explaining difficult topics to new learners.
- Flutter Clutter – This site has a collection of tutorials, troubleshooting and answers to common questions regarding app development with Flutter. The owner uploads tutorials and flutter basics on a regular basis.
Architecture Tutorials
- Flutter app architecture 101 – This post introduces three different approaches to the architecture: Vanilla, Scoped Model, BLoC.
- Architect your app using Provider and Stream – If you want an introduction to the different state management techniques using Provider and Stream, read this blog post.
- flutter_clean_architecture – A Flutter package that makes it easy and intuitive to implement Uncle Bob’s Clean Architecture in Flutter.
- Getting Started with the BLoC Pattern – BLoC makes it easy to separate the presentation layer from the business logic, making your code fast, easy to test, and reusable.
- starter_architecture_flutter_firebase – This is a reference architecture demo that can be used as the foundation for Flutter apps using Firebase (or other streaming APIs).
- State Management With Provider – In this tutorial, you’ll learn the ins and outs of managing states with Provider by creating a currency exchange app with architecture, Provider implementation, and app state management.
Flutter for Games
- Making Games with Flutter – A collection of libraries helps making a game with Dart and Flutter.
Must-Have Libraries
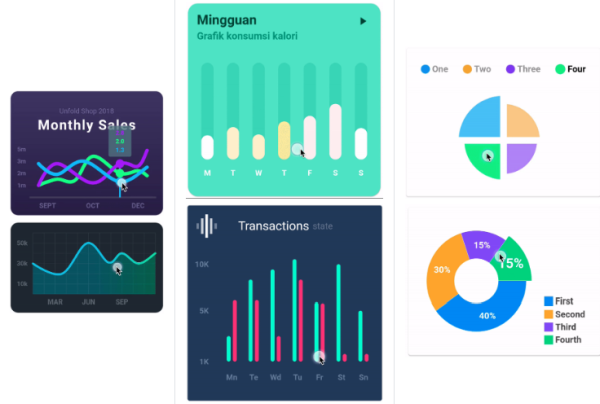
- Flutter Plugins: This repository consists of plugins developed by official Flutter Team. They handle backbone features of a mobile app such as Alarm Manager, Firrbase service, WebView, Video Player, Maps, etc.
- win32 – A package that wraps some of the most common Win32 API calls using FFI to make them accessible to Dart code without requiring a C compiler or the Windows SDK.
- fl_chart – A library to draw fantastic charts. It has several useful graphs, such as bar, line and pie charts.
- Charts – This plugin is developed by Google team. It supports over 50 types of charts and designs.
- dio – A powerful Http client for Dart, which supports Interceptors, Global configuration, FormData, Request Cancellation, File downloading, Timeout etc.
- ezanimation – An easy to use animation library. With EzAnimation, there is no need to burden your code with TickerProviders, the complicated logic of curves, different components of animation, etc.
- rxdart – RxDart is a reactive functional programming library for Google Dart based on ReactiveX. RxDart adds functionality from the reactive extensions specification on top of Dart’s Streams API.
- intro_slider – Flutter Intro Slider is a flutter plugin that helps you make a cool intro for your app.
- path_provider – Find commonly used locations on the filesystem on both Android and iOS.
- cached_network_image – It shows and caches images downloaded from internet. This widget now uses builders for the placeholder and error widget and uses sqflite for cache management.
- font_awesome_flutter – Use Font Awesome icons in your app.
- time.dart – Type-safe DateTime and Duration calculations, powered by extensions.
- google_fonts – The google_fonts package for Flutter allows you to easily use any of the 977 fonts (and their variants) from fonts.google.com in your Flutter app.
- flutter_svg – This package allows to draw SVG (and some Android VectorDrawable (XML)) files on a Flutter Widget.
- flare_flutter – Flare offers powerful realtime vector design and animation for app and game designers alike. This library is Flutter runtime for Flare, depends on flare_dart.
- clean_settings – Creating a settings screen requires the same boiler plate code over and over. This library aims to provide sane defaults while creating a setting screen.
- battery_plus – Access various information about the battery of the device the app is running on.
- connectivity_plus – This allows Flutter apps to discover network connectivity and configure themselves accordingly. It can distinguish between cellular vs WiFi connection.
- device_info_plus – Get current device information from within the Flutter application.
- package_info_plus – Provides an API for querying information about an application package.
- sensor_plus – Access the accelerometer and gyroscope sensors.
- share_plus – A Flutter plugin to share content from your Flutter app via the platform’s share dialog.
- android_alarm_manager_plus – Accessing the Android AlarmManager service, and running Dart code in the background when alarms fire.
- android_intent_plus – Launch arbitrary intents when the platform is Android.
- Sqflite: SQLite Flutter plugin. It supports transactions and batches, automatic version managment during open, helpers for insert/query/update/delete queries, and DB operation executed in a background thread on iOS and Android.
- shared_preferences: Wraps NSUserDefaults (on iOS) and SharedPreferences (on Android), providing a persistent store for simple data.
- Login: This supports login with FaceID, TouchID, and Fingerprint Reader on Android.
- Flutter Location: This plugin handles getting location on Android and iOS. It also provides callbacks when location is changed.
- QR Code Reader: QR Code reader via camera
- Battery: This plugin allows app to access to various information about the battery.
- Zebra EMDK: Use Zebra EMDK to access Barcode Scanner API.
- Proximity Sensor Plugin: A plugin to access the proximity sensor of your device
- Geolocation: This is a fully featured geolocation plugin which supports Manual and automatic location permission management, current one-shot location, continuous location updates with foreground and background options.
- Local Notifications: This help dipslay local notifications. It can display basic notifications, schedule when notifications should appear, show a notification periodically or at a specified time.
- Google Mobile Vision: This is an implementation of Google Mobile Vision.
- Offline: A tidy utility to handle offline/online connectivity.
- 3D Object: Renders a wavefront .obj on to a canvas.
- Speech Recognition: A flutter plugin to use the speech recognition iOS10+ / Android 4.1+
- Chewie: The video_player plugin provides low-level access to video playback. Chewie uses the video_player under the hood and wraps it in a friendly Material or Cupertino UI.
- Audio Plugin: Play audio files.
Starter Kits / Templates
Templates
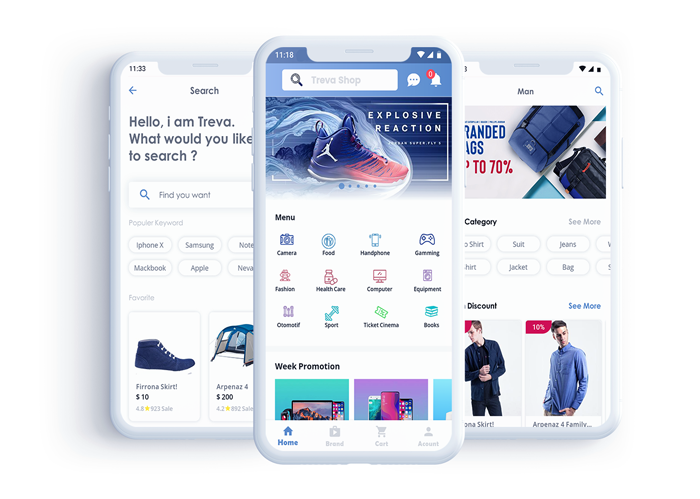
E-Commerce UI kit can be used for e-commerce applications in android and ios devices. It contains 32 Screens with a different type of UI, E-Commerce UI kit can save your time to code all Front end layout.
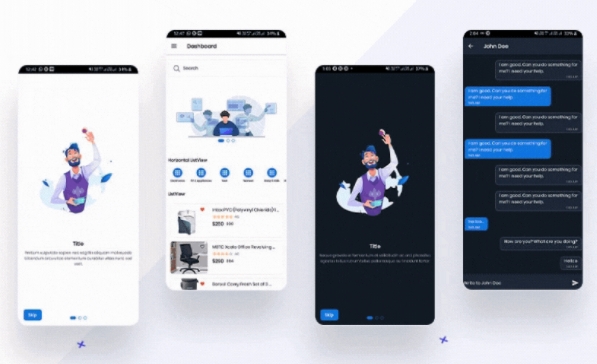
It is the ultimate library of Flutter UI app templates which is perfect for business like ecommerce store app, bus and travel booking app, hotel booking app, grocery delivery and food delivery app, online education and online learning app, digital wallet app, health and gym app, movie streaming and much more
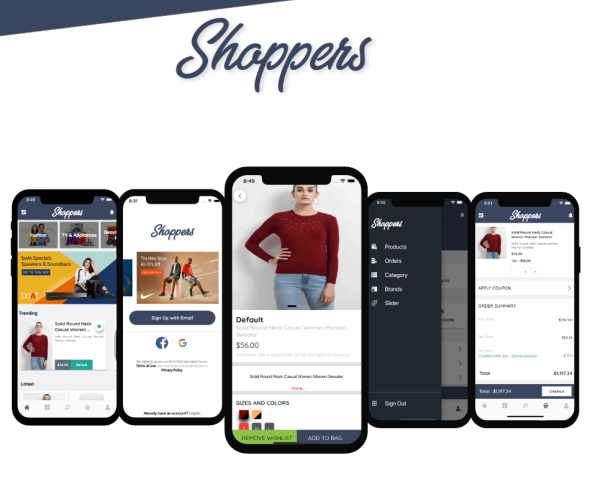
A Flutter E-Commerce app created using Firebase, Stripe and Razorpay.
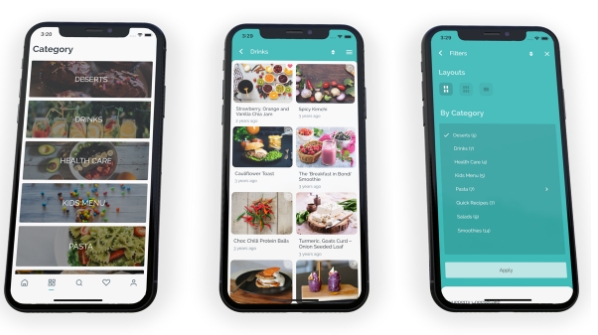
This kit can turn your WordPress website into a news app.
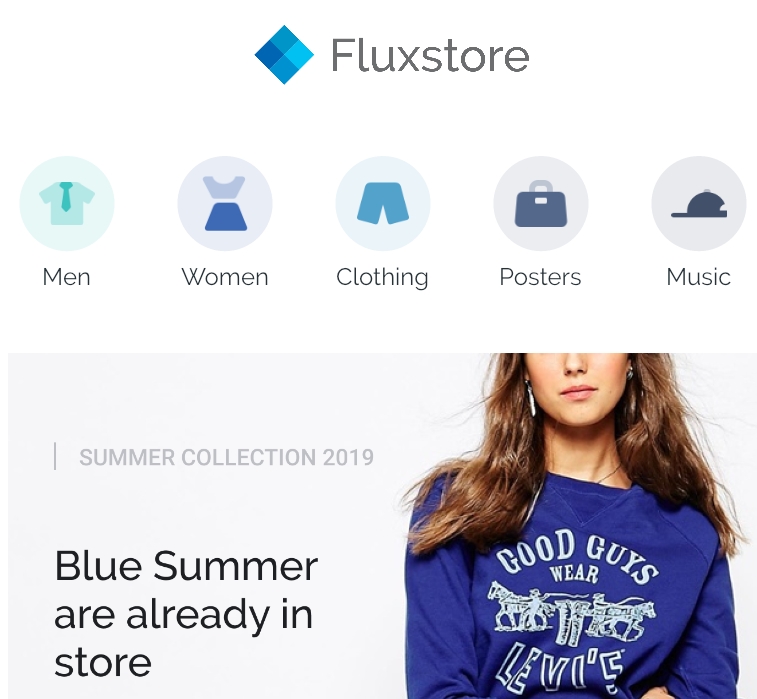
Fluxstore WooCommerce is an universal e-commerce app inspired by the Flutter framework. If your business has already had the website that built based on WooCommerce, then it is easy to integrate with Fluxstore by just a few steps, and quickly release the final app to both Appstore and Google Play store
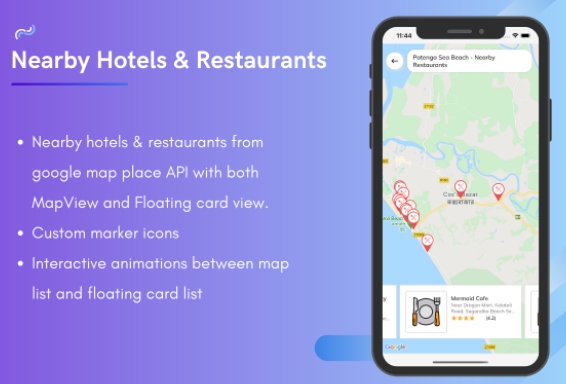
Travel Hour is a complete travel guide app based on a country, it has also an admin panel. The app uses Google maps and Its APIs to get nearby data like hotels and restaurants and show routes between the source and destination. The template contains 50+ screens.
Starter Kits
- Flutter App and UI Templates – This page list all common layout widgets used in Flutter, and a collection of template boilerplate for certain designs.
- first_app – Various elements and features are put together into this single app. It offers global UI theme, separation of business logic in models and providers, use of scoped_model for app state management, unit test, custom icons for both iOS and Android, Firebase Analytics & Crashlytics for tracking, and many more.
- flutter-starter-kit – A Flutter starter application that utilizes the BLoC Pattern.
- Flutter Starter – This is a starter-kit for production-level apps, including folder structure, API call, style guide, state management, router, and testing.
Tools
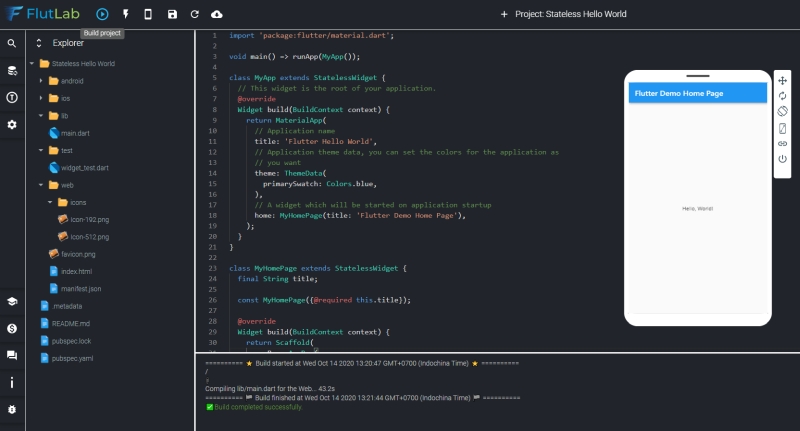
- FlutterFlow – FlutterFlow is an app visual builder which helps building Flutter applications. Just drag and drop the required widgets then download source code to your computer or publish it to app stores.
- FlutLab – This is a playground for writting Flutter app. It offers an emulator to see the output as well.
- FigmaToCode – Figma To Code can generate responsive layouts in Tailwind and Flutter from Figma design.
- AppWrite – This is an end-to-end backend server that is aiming to abstract the complexity of common, complex, and repetitive tasks required for building a modern app.
- cpainterhelper – A simple script that lets you convert adobe illustrator paths to code compatible with Flutter’s CustomPainter.
- Repix.app – Create beautiful screenshots for App Store and Play Store.
- Previewed.app – Create intro videos, screenshots for your app and for FREE.
Tips
Enable CanvasKit / Skia in Flutter Web
flutter run -d chrome --dart-define=FLUTTER_WEB_USE_SKIA=true flutter build web --dart-define=FLUTTER_WEB_USE_SKIA=true --release
Quickly create Stateless and Stateful widgets
Android Studio can generate boilerplate for these two widgets if users type stless
and stful
to get Autocomplete Suggestion.
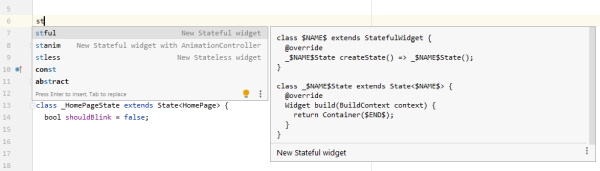
Use const before Constructor to avoid rebuilding a widget
If you want to prevent unwanted widget rebuilds always use const constructor.
Container( width: double.infinity, height: 300, decoration: const BoxDecoration( borderRadius: BorderRadius.only( bottomLeft: const Radius.circular(10.0), bottomRight: const Radius.circular(10.0))), child: Text('Container') )
Spread Operator
The Dart 2.3 introduced Spread operator (...
), which is useful for nested conditional UI Widget.
var a = [0,1,2]; var b = [...a,3]; print(b); //[0,1,2,3]
bool shouldBlink = true; Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ Text('User choose condition below'), if (shouldBlink) ...[ Center( child: BlinkText( 'Blink Animation', style: TextStyle(fontSize: 24), beginColor: Colors.red, endColor: Colors.blueAccent, textDirection: TextDirection.ltr, duration: Duration(seconds: 1), ), ), ] else ...[ Text('No blinking allowed') ] ], )
Use Cascade Operator (..) to chain properties and methods
Account() ..username = 'TLTemplates' ..password = $hashed ..setActive();
Check null with ??
operator
??
operator can be used to check if a variable is null and set a default value for that variable.
final endColor = widget.endColor ?? Colors.transparent;
Use SafeArea widget to avoid phone’s notch and app bar
SafeArea widget can keep notification bars and phone notches from overlapping with your app’s UI.
Use Spacer widget to responsively adding space between widgets
Putting a Spacer widget among widgets will help create equal spaces among them. These spaces are resized according to current screen size as well.
Row( children: [ Text('Text'), Spacer(), Text('Text'), Spacer(), Text('Text'), Spacer(), Text('Text'), Spacer(), Text('Text'), ], ),
Use pre-defined TextStyle
Styling individual Text widget is a repetitive task. You can use Theme.of(context).textTheme
to use the pre-defined text style.
Text('headline', style: Theme.of(context).textTheme.headline1,), Text('Body Text', style: Theme.of(context).textTheme.bodyText1,),
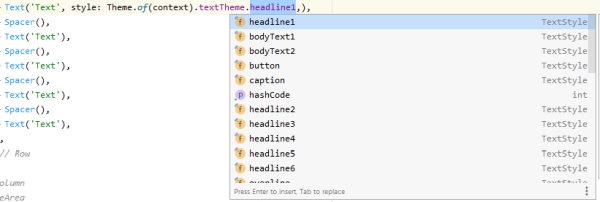
Bulk declaration
Variables of the same type can be defined all at once.
double width,height,degree,fontSize; int age = 30,size = 24;
Delay an action
An object of Future class represents a delayed computation.
Future.delayed(Duration(seconds: 3), () { print('This is executed after 3 seconds'); });
Flutter logs
There is a command to log the output of the running flutter app, which is flutter logs
. You can execute this command line in multiple terminal windows/tabs in Android studio, which allows you to look back in the history of the log output of the previous app run.
That’s a great overview of helpful tips and resources for Flutter beginners, thank you.
Would you mind adding my website to the list of resources as well? I upload tutorials and flutter basics on a regular basis: https://www.flutterclutter.dev/
Can you add this Youtube Playlist also ??
Flutter – WooCommerce – WordPress – Series
https://www.youtube.com/playlist?list=PL7zgwanvi8_MZGiW8wSL1k7y_92P7UMW6
I added your playlist. If you have any resources, you can introduce them to us by using the URL in the menu.
Awesome content keep updating those tools and libraries and also add some new.