This post will introduce some ways to make a Flutter widget center horizontally and vertically within a screen.
Table of Contents
Use Center widget
Center is a widget that make its child center-aligned within itself. Center widget will be as big as possible if its dimensions are constrained and widthFactor
and heightFactor
are null
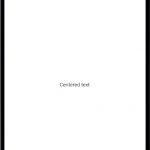
Scaffold( appBar: AppBar( title: Text('Home'), ), backgroundColor: Colors.white, body: SafeArea( child: Center(child: Text('Centered text')), ), );
Use Align
Align widget aligns its child within itself and optionally sizes itself based on the child’s size. Its alignment
determine how to align its child. alignment: Alignment.center
makes its child align center vertically and horizontally.
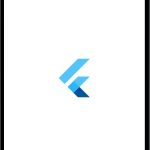
Container( width: double.infinity, height: double.infinity, child: Align( alignment: Alignment.center, child: FlutterLogo( size: 120, ), ), )
Use Column
Column widget displays its children in a vertical array. It has 2 properties which help align its children, which are mainAxisAlignment
and crossAxisAlignment
. The former one determines how its children should be placed along the main axis, the later uses cross axis.
If we wrap a Column within a Container with max width and height, we can set Column’s children to center aligned by applying mainAxisAlignment: MainAxisAlignment.center
and crossAxisAlignment: CrossAxisAlignment.center
.
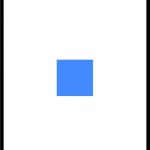
Container( width: double.infinity, height: double.infinity, child: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ Container( width: 100, height: 100, color: Colors.blueAccent, ) ], ), )
Use Row
Row displays its children in a horizontal array. We can apply Row widget to solve this problem like we apply Column, but only need mainAxisAlignment: MainAxisAlignment.center
property.
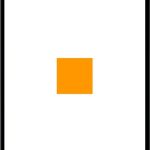
Container( width: double.infinity, height: double.infinity, child: Row( mainAxisAlignment: MainAxisAlignment.center, children: [ Container( width: 100, height: 100, color: Colors.orange, ) ], ), )