We attach a curve ClipPath at the bottom of AppBar to make it a part of the AppBar for the look and feel.
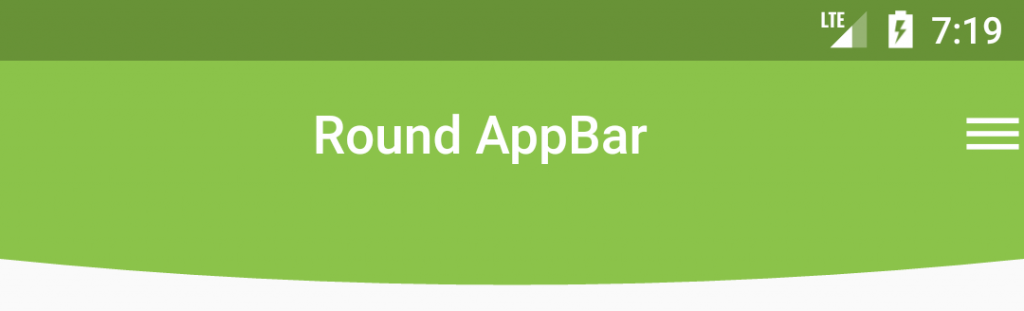
class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Center(child: Text('Round AppBar')), backgroundColor: Colors.lightGreen, elevation: 0, actions: [Icon(Icons.dehaze_sharp)], ), body: SafeArea( child: Column( children: [ ClipPath( clipper: RoundShape(), child: Container( height: 40, color: Colors.lightGreen, ), ), Center( child: Text('Body'), ), ], )), ); } } class RoundShape extends CustomClipper<Path> { @override getClip(Size size) { double height = size.height; double width = size.width; double curveHeight = size.height / 2; var p = Path(); p.lineTo(0, height - curveHeight); p.quadraticBezierTo(width / 2, height, width, height - curveHeight); p.lineTo(width, 0); p.close(); return p; } @override bool shouldReclip(CustomClipper oldClipper) => true; }