The idea of autoloading classes in PHP is not a new one. It’s been around as long as PHP has been, and it’s always been a bit of a dark art for those who don’t have the luxury of having written their own framework or engine from scratch. But now that more people are migrating to frameworks like Laravel, Symfony, or Zend Framework 2+, they’re getting exposed to autoloading whether they want to or not!
In PHP, you can load classes without calling them explicitly using what is called autoloading. This means that the class name is passed to a function which will do all of the work for you. The autoloader looks for files with a specific naming convention and loads them automatically when it finds one. If none are found, an exception will be thrown informing you of this fact.
There are many reasons to write a custom class or library to carry out certain tasks in Laravel. We need to include the file to autoload to use it later in a controller.
Laravel doesn’t have a fixed folder to store library files starting from Laravel 5. We can create a new folder under App folder called Libraries (App/Libraries
).
Open composer.json and add the newly created folder “app/Libraries” to autoload list.
"autoload": { "psr-4": { "App\\": "app/" }, "classmap": [ "database/seeds", "database/factories", "app/Libraries" ] },
Full composer.json file:
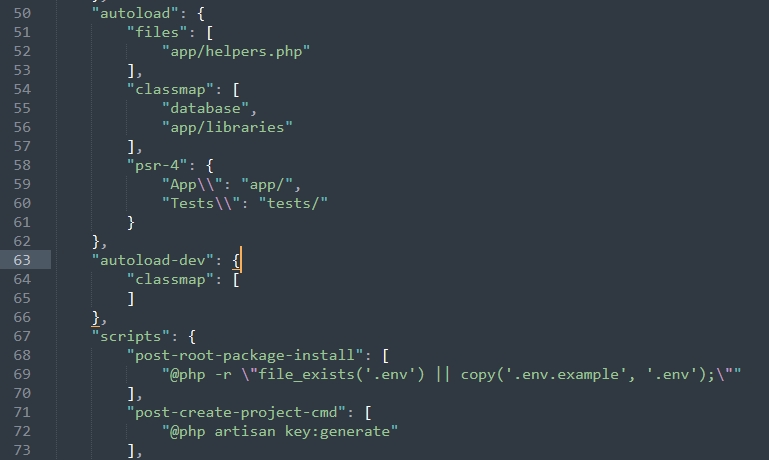
{ "name": "laravel/laravel", "type": "project", "description": "The Laravel Framework.", "keywords": [ "framework", "laravel" ], "license": "MIT", "require": { "php": "^7.2.5|^8.0", "fideloper/proxy": "^4.4", "fruitcake/laravel-cors": "^2.0", "guzzlehttp/guzzle": "^6.3.1|^7.0.1", "laravel/framework": "^7.29", "laravel/sanctum": "^2.8", "laravel/tinker": "^2.5", "laravel/ui": "^2.5", "laravelcollective/html": "^6.2", "spatie/laravel-permission": "^3.18" }, "require-dev": { "facade/ignition": "^2.0", "fakerphp/faker": "^1.9.1", "mockery/mockery": "^1.3.1", "nunomaduro/collision": "^4.3", "phpunit/phpunit": "^8.5.8|^9.3.3" }, "config": { "optimize-autoloader": true, "preferred-install": "dist", "sort-packages": true }, "extra": { "laravel": { "dont-discover": [] } }, "autoload": { "psr-4": { "App\\": "app/" }, "classmap": [ "database/seeds", "database/factories", "app/Libraries" ] }, "autoload-dev": { "psr-4": { "Tests\\": "tests/" } }, "minimum-stability": "dev", "prefer-stable": true, "scripts": { "post-autoload-dump": [ "Illuminate\\Foundation\\ComposerScripts::postAutoloadDump", "@php artisan package:discover --ansi" ], "post-root-package-install": [ "@php -r \"file_exists('.env') || copy('.env.example', '.env');\"" ], "post-create-project-cmd": [ "@php artisan key:generate --ansi" ] } }
Then run composer dump-autoload
after saving the composer.json file’s changes.
Under App\Libraries, we can create any classes and we can call them later in other controllers.
<?php namespace App\Libraries; use Illuminate\Support\Facades\Http; use App\User; use App\Order; class Utils { public static function orderUsers($order, $bold = true){ $names = $this->usersOfOrder($order); $short_id = isset($order->short_id) ? $order->short_id : substr($order->order_id, 0, 8); $short_id = $bold ? "<b>{$short_id}</b>" : $short_id; if(!empty($names)){ return $short_id.' ('.implode(', ', $names).')'; } else { return $short_id; } } }